boost库json 的所有用法
时间: 2024-05-03 16:21:11 浏览: 176
Boost库的JSON模块提供了一组用于处理JSON数据的工具和类。以下是Boost库JSON的常见用法:
1. 解析JSON数据
使用json::parse()函数将JSON字符串解析为JSON对象:
```c++
#include <boost/json.hpp>
#include <iostream>
int main()
{
std::string json_str = R"(
{
"name": "John",
"age": 30,
"is_student": true,
"address": {
"street": "123 Main St",
"city": "Anytown",
"state": "CA"
},
"scores": [85, 90, 92]
}
)";
boost::json::value json_obj = boost::json::parse(json_str);
std::cout << json_obj << std::endl;
return 0;
}
```
2. 获取JSON对象的值
使用json::value的成员函数获取JSON对象的键和值:
```c++
#include <boost/json.hpp>
#include <iostream>
int main()
{
std::string json_str = R"(
{
"name": "John",
"age": 30,
"is_student": true,
"address": {
"street": "123 Main St",
"city": "Anytown",
"state": "CA"
},
"scores": [85, 90, 92]
}
)";
boost::json::value json_obj = boost::json::parse(json_str);
std::string name = json_obj.at("name").as_string().data();
int age = json_obj.at("age").as_int64();
bool is_student = json_obj.at("is_student").as_bool();
std::string street = json_obj.at("address").as_object().at("street").as_string().data();
int score1 = json_obj.at("scores").as_array()[0].as_int64();
std::cout << "Name: " << name << std::endl;
std::cout << "Age: " << age << std::endl;
std::cout << "Is student: " << is_student << std::endl;
std::cout << "Street: " << street << std::endl;
std::cout << "Score 1: " << score1 << std::endl;
return 0;
}
```
3. 构造JSON对象
使用json::value构造函数或json::value的成员函数构造JSON对象:
```c++
#include <boost/json.hpp>
#include <iostream>
int main()
{
boost::json::object obj {
{"name", "John"},
{"age", 30},
{"is_student", true},
{"address", {
{"street", "123 Main St"},
{"city", "Anytown"},
{"state", "CA"}
}},
{"scores", {85, 90, 92}}
};
boost::json::value json_obj(obj);
std::cout << json_obj << std::endl;
return 0;
}
```
4. 修改JSON对象的值
使用json::value的成员函数修改JSON对象的值:
```c++
#include <boost/json.hpp>
#include <iostream>
int main()
{
std::string json_str = R"(
{
"name": "John",
"age": 30,
"is_student": true,
"address": {
"street": "123 Main St",
"city": "Anytown",
"state": "CA"
},
"scores": [85, 90, 92]
}
)";
boost::json::value json_obj = boost::json::parse(json_str);
json_obj.as_object()["name"] = "Jane";
json_obj.as_object()["age"] = 35;
json_obj.as_object()["is_student"] = false;
json_obj.as_object()["address"].as_object()["street"] = "456 Elm St";
json_obj.as_object()["scores"].as_array()[1] = 95;
std::cout << json_obj << std::endl;
return 0;
}
```
5. 序列化JSON对象
使用json::to_string()函数将JSON对象序列化为JSON字符串:
```c++
#include <boost/json.hpp>
#include <iostream>
int main()
{
std::string json_str = R"(
{
"name": "John",
"age": 30,
"is_student": true,
"address": {
"street": "123 Main St",
"city": "Anytown",
"state": "CA"
},
"scores": [85, 90, 92]
}
)";
boost::json::value json_obj = boost::json::parse(json_str);
std::string serialized_json = boost::json::to_string(json_obj);
std::cout << serialized_json << std::endl;
return 0;
}
```
阅读全文
相关推荐





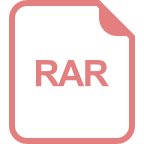












