java解析占位符${}
时间: 2023-09-07 12:14:42 浏览: 90
在Java中,可以使用占位符`${}`来创建模板字符串。这种方式通常用于配置文件中,可以在配置文件中定义占位符,然后在程序运行时动态替换这些占位符。
要解析`${}`占位符,可以使用Java自带的Properties类。Properties类是一个键值对的集合,通常用于读取和写入Java的配置文件。
假设我们有一个配置文件config.properties,其中定义了一个占位符${name}:
```
greeting=Hello, ${name}!
```
我们可以使用如下代码读取该配置文件,并替换其中的占位符:
```java
Properties props = new Properties();
props.load(new FileInputStream("config.properties"));
String greeting = props.getProperty("greeting");
String name = "John";
String message = greeting.replace("${name}", name);
System.out.println(message);
```
在上面的代码中,我们先读取config.properties文件,并获取其中的greeting属性。然后,我们将name变量的值替换到占位符${name}中,并输出结果。
需要注意的是,如果配置文件中存在多个占位符,需要依次替换每个占位符,否则可能会出现解析错误。
相关问题
java解析占位符${}中的内容
在Java中,可以使用正则表达式来解析占位符`${}`中的内容。具体实现方式如下:
```java
Pattern pattern = Pattern.compile("\\$\\{(.+?)\\}");
String input = "Hello, ${name}! You are ${age} years old.";
Matcher matcher = pattern.matcher(input);
while (matcher.find()) {
String placeholder = matcher.group(0);
String key = matcher.group(1);
// 根据key获取对应的值,并替换占位符
String value = getValueByKey(key);
input = input.replace(placeholder, value);
}
System.out.println(input);
```
在上面的代码中,我们首先使用正则表达式`\$\{(.+?)\}`来匹配占位符`${}`中的内容。其中,`\$`表示转义字符,用来匹配`${}`中的`$`字符;`\{`和`\}`分别表示左右花括号,用来匹配`${}`中的内容。
然后,我们使用Matcher对象来匹配输入字符串中所有的占位符,并依次替换每个占位符。在替换时,我们需要根据占位符中的key值来获取对应的value值,具体实现方式可以根据具体需求而定。
需要注意的是,如果输入字符串中存在多个相同的占位符,需要依次替换每个占位符,否则可能会出现解析错误。
Java 解析word中的占位符
要解析Word中的占位符,你需要使用一些Java库,如Apache POI和Docx4j。这些库可以读取Word文档并让你访问其中的内容,包括占位符。
以下是使用Apache POI解析Word中占位符的示例代码:
```
import org.apache.poi.xwpf.usermodel.*;
import java.io.*;
public class PlaceholderParser {
public static void main(String[] args) throws Exception {
// Load the Word document
FileInputStream fis = new FileInputStream("template.docx");
XWPFDocument doc = new XWPFDocument(fis);
// Iterate over the paragraphs in the document
for (XWPFParagraph paragraph : doc.getParagraphs()) {
// Iterate over the runs in the paragraph
for (XWPFRun run : paragraph.getRuns()) {
// Check if the run contains a placeholder
if (run.getText(0).contains("{{placeholder}}")) {
// Replace the placeholder with your own text
run.setText(run.getText(0).replace("{{placeholder}}", "Hello, world!"));
}
}
}
// Save the modified document
FileOutputStream fos = new FileOutputStream("output.docx");
doc.write(fos);
fos.close();
doc.close();
}
}
```
在这个示例中,我们打开名为"template.docx"的Word文档,遍历其所有段落和运行,查找包含"{{placeholder}}"的运行。如果找到了占位符,我们将使用"Hello, world!"替换它。最后,我们将修改后的文档保存到名为"output.docx"的文件中。
你可以根据需要更改代码,以便解析不同类型的占位符。
阅读全文
相关推荐
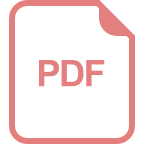
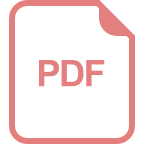
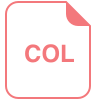

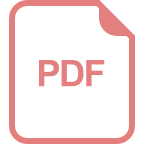
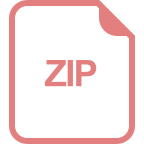
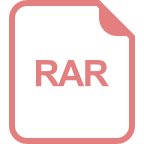
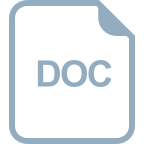
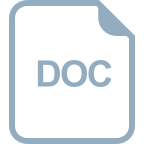
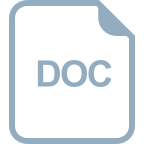
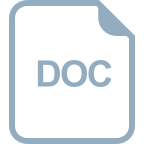
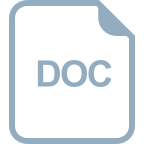
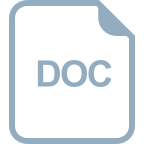
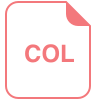
