编写一个Cube组件,入参为num, 当num=3时,输出表格效果为: 1 1 1 2 2 2 3 3 3 当num=4时,输出表格效果为: 1 1 1 1 2 2 2 2 3 3 3 3 4 4 4 4 以此类推。 要求: 根据代码样例文件(index.vue和Cube.vue)进行修改 在父组件(index.vue)实现输入框,输入或修改数字后自动刷新生成表格 子组件(Cube.vue)中判断参数是否合法(数字且在[1,10]之间),如果不合法,通知父组件,父组件背景变为红色,合法则恢复原来颜色
时间: 2023-12-10 15:41:23 浏览: 59
以下是可以实现题目要求的代码:
index.vue:
```
<template>
<div :style="{background: bgColor}">
<input v-model.number="num" @input="checkNum" />
<cube :num="num" @valid="isValid" />
</div>
</template>
<script>
import Cube from "./Cube.vue";
export default {
name: "Index",
components: {
Cube,
},
data() {
return {
num: 3,
bgColor: "white",
};
},
methods: {
checkNum() {
if (this.num < 1 || this.num > 10 || isNaN(this.num)) {
this.bgColor = "red";
} else {
this.bgColor = "white";
}
},
isValid(isValid) {
if (!isValid) {
this.bgColor = "red";
} else {
this.bgColor = "white";
}
},
},
};
</script>
```
Cube.vue:
```
<template>
<div>
<table>
<tbody>
<tr v-for="row in rows">
<td v-for="num in numList">{{ num }}</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
export default {
name: "Cube",
props: {
num: {
type: Number,
required: true,
validator: (value) => {
return value >= 1 && value <= 10 && !isNaN(value);
},
},
},
computed: {
numList() {
return Array.from({ length: this.num }, (_, i) => i + 1);
},
rows() {
return Array.from({ length: this.num }, () => null);
},
},
created() {
if (!this.$props.validator(this.num)) {
this.$emit("valid", false);
} else {
this.$emit("valid", true);
}
},
};
</script>
```
在父组件中,我们使用了 `v-model.number` 对输入框的值进行绑定,并在输入框的 `@input` 事件上调用 `checkNum` 方法检查输入框中的值是否合法,如果不合法,则将父组件的背景颜色设置为红色。
在子组件中,我们使用了 `props` 来接收父组件传递过来的参数,并使用 `validator` 对传递过来的值进行验证。如果验证不通过,我们就使用 `$emit` 方法向父组件发送 `valid` 事件,并传递 `false`,表示当前传递过来的值不合法。
在子组件的 `computed` 中,我们通过 `Array.from` 和 `length` 属性来生成一个长度为 `num` 的数组,并使用 `v-for` 来将数组中的每个元素渲染成表格中的一行。在每一行中,我们又使用 `v-for` 将 `numList` 数组中的每个元素渲染成表格中的一列。
最后,在子组件的 `created` 钩子函数中,我们对传递过来的参数进行验证并向父组件发送 `valid` 事件,以便在父组件中更新背景颜色。
阅读全文
相关推荐
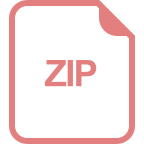
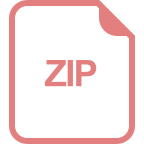
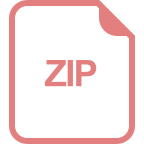
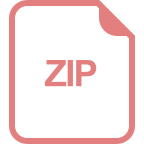
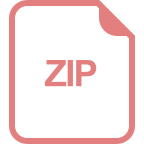
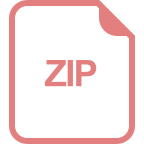
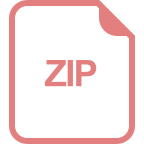
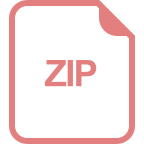
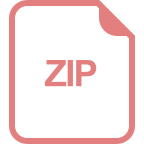
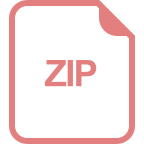
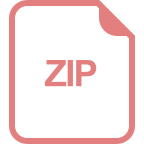
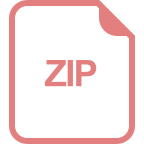
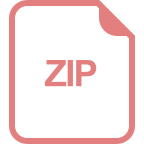
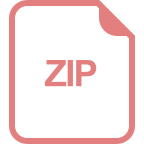
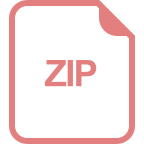
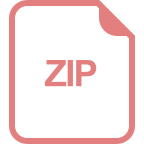
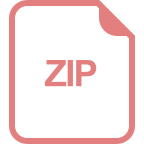