vue 阻止浏览器刷新按钮 刷新页面
时间: 2023-09-29 15:06:54 浏览: 54
在 Vue 中,你可以通过监听 `beforeunload` 事件来阻止浏览器刷新按钮刷新页面。具体实现如下:
```javascript
export default {
mounted() {
window.addEventListener('beforeunload', this.beforeWindowUnload)
},
beforeDestroy() {
window.removeEventListener('beforeunload', this.beforeWindowUnload)
},
methods: {
beforeWindowUnload(event) {
// 取消默认事件
event.preventDefault()
// Chrome 需要返回值
event.returnValue = ''
}
}
}
```
在 `mounted` 钩子中,我们注册了 `beforeunload` 事件,并指定了回调函数 `beforeWindowUnload`。在回调函数中,我们通过调用 `preventDefault` 方法和设置 `event.returnValue` 的值来阻止浏览器刷新按钮刷新页面。在 `beforeDestroy` 钩子中,我们移除了 `beforeunload` 事件监听器,以免造成内存泄漏。
相关问题
vue-admin-template工程,点击浏览器刷新按钮时刷新很慢
这个问题可能与webpack-dev-server的配置有关。可以尝试增加以下配置项来提高刷新速度:
1. 在webpack.dev.conf.js中增加以下内容:
```javascript
devServer: {
clientLogLevel: 'warning',
hot: true,
contentBase: false, // since we use CopyWebpackPlugin.
compress: true,
host: 'localhost',
port: 8080,
open: false,
overlay: { warnings: false, errors: true },
publicPath: '/',
quiet: true,
watchOptions: {
poll: true,
},
},
```
2. 在package.json中增加以下内容:
```json
"config": {
"webpackDevServer": {
"disableHostCheck": true
}
},
```
以上两个配置项可以让webpack-dev-server在进行热更新时更加快速,同时解决一些可能出现的网络问题导致的刷新缓慢问题。
vue3点击按钮刷新图片
你可以使用Vue3的响应式数据和v-bind指令来实现点击按钮刷新图片的功能。具体实现方法如下:
1. 在Vue3组件的data属性中定义一个变量,用于存储图片的URL,例如:
```
data() {
return {
imageUrl: 'https://example.com/image.jpg'
}
}
```
2. 在组件的模板中使用v-bind指令将imageUrl绑定到img标签的src属性上,例如:
```
<template>
<div>
<img v-bind:src="imageUrl" alt="示例图片">
<button @click="refreshImage">刷新图片</button>
</div>
</template>
```
3. 定义一个方法refreshImage,用于在点击按钮时更新imageUrl变量的值,例如:
```
methods: {
refreshImage() {
// 生成一个随机数作为查询参数,防止浏览器缓存
const randomQuery = Math.random().toString(36).substring(7);
this.imageUrl = `https://example.com/image.jpg?${randomQuery}`;
}
}
```
4. 点击按钮时,调用refreshImage方法即可刷新图片。
相关推荐
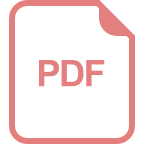












