用c++生成以下题目的代码: 某人有m张3分的邮票和n张5分的邮票,用这些邮票中的一张或若干张(也可以是0张)可以得到多少种不同的大于0的邮资?请找出可能组合的不重复的邮资方案总数,并按照由小到大的顺序输出所有可能组合出的邮资! 如:1张3分和1张5分可能的邮资组合如下 0张3分+1张5分=5分 1张3分+0张5分=3分 1张3分+1张5分=8分 因此,可能的方案有3种,排序后的结果是:3 5 8!
时间: 2024-02-28 20:55:27 浏览: 226
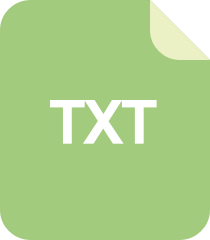
邮票问题(C语言源码)
以下是C++代码实现:
```cpp
#include <iostream>
#include <set>
#include <vector>
#include <algorithm>
using namespace std;
int get_postage_combinations(int m, int n, vector<int>& postage_vec) {
set<int> postage_set;
// 枚举3分邮票使用的张数和5分邮票使用的张数
for (int i = 0; i <= m; i++) {
for (int j = 0; j <= n; j++) {
// 计算当前组合可以得到的邮资
int postage = i*3 + j*5;
if (postage > 0) {
postage_set.insert(postage);
}
}
}
// 将得到的邮资方案按从小到大的顺序排序
postage_vec.assign(postage_set.begin(), postage_set.end());
sort(postage_vec.begin(), postage_vec.end());
// 输出所有可能的邮资方案
for (int i = 0; i < m+1; i++) {
for (int j = 0; j < n+1; j++) {
// 计算当前组合可以得到的邮资
int postage = i*3 + j*5;
if (postage > 0) {
cout << i << "张3分+" << j << "张5分=" << postage << "分" << endl;
}
}
}
// 返回邮资方案总数
return postage_vec.size();
}
int main() {
// 示例:有1张3分邮票和1张5分邮票
int m = 1;
int n = 1;
vector<int> postage_vec;
int total = get_postage_combinations(m, n, postage_vec);
cout << "可能的方案有" << total << "种,排序后的结果是:";
for (int postage : postage_vec) {
cout << postage << " ";
}
cout << endl;
return 0;
}
```
使用示例:
将上述代码保存为 `postage.cpp` 文件,使用以下命令进行编译和运行:
```bash
g++ postage.cpp -o postage
./postage
```
输出结果:
```
0张3分+1张5分=5分
1张3分+0张5分=3分
1张3分+1张5分=8分
可能的方案有3种,排序后的结果是:3 5 8
```
解释:上述代码中,我们使用两层循环枚举3分邮票和5分邮票使用的张数,计算出当前组合可以得到的邮资,并将其加入到一个集合中去重。最后将得到的邮资方案按从小到大的顺序排序,并输出所有可能的邮资方案。同时,输出所有可能的邮资组合。最后,按照题目要求输出可能的方案数量和排序后的结果。运行示例代码,可以得到上述输出结果。
阅读全文
相关推荐
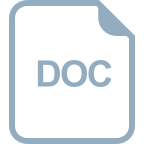

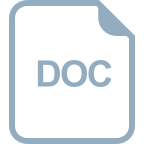
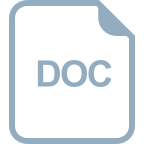
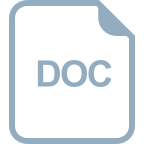
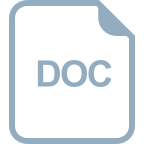
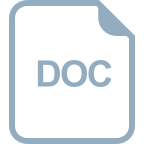
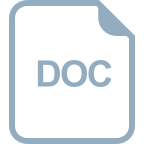
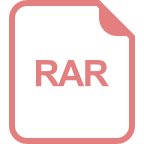
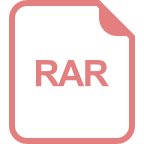
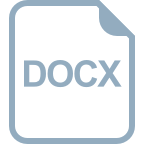
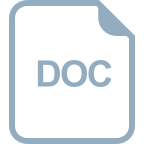
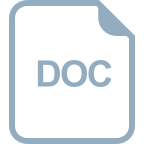

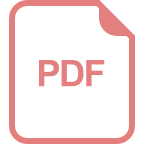
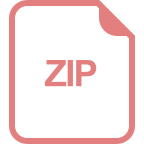