c代码如何实现LZSS算法
时间: 2024-05-09 12:15:39 浏览: 300
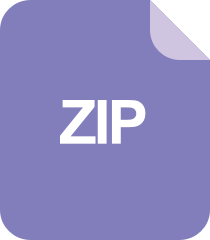
lzss压缩解压缩算法。
LZSS 算法是一种常用的无损压缩算法,下面是一个 C 语言实现 LZSS 算法的示例代码:
```c
#include <stdio.h>
#include <string.h>
#define WINDOW_SIZE 4096
#define LOOK_AHEAD_SIZE 18
#define MIN_MATCH 3
unsigned char window[WINDOW_SIZE];
unsigned char lookAheadBuffer[LOOK_AHEAD_SIZE];
int lookAheadSize;
void slideWindow(int n)
{
memcpy(window, window + n, WINDOW_SIZE - n);
memset(window + WINDOW_SIZE - n, 0, n);
}
int searchMatch(int start, int *matchPos)
{
int longestMatch = 0;
for (int i = 0; i < WINDOW_SIZE - start; i++) {
int len = 0;
while (len < LOOK_AHEAD_SIZE && lookAheadBuffer[len] == window[start + i + len]) {
len++;
}
if (len > longestMatch) {
longestMatch = len;
*matchPos = start + i;
}
if (longestMatch == LOOK_AHEAD_SIZE) {
break;
}
}
return longestMatch;
}
void outputLiteral(unsigned char ch)
{
printf("%d ", ch);
}
void outputCode(int offset, int length)
{
printf("(%d,%d) ", offset, length);
}
void compress(unsigned char *data, int size)
{
int i = 0;
while (i < size) {
if (lookAheadSize < LOOK_AHEAD_SIZE && i < size) {
lookAheadBuffer[lookAheadSize++] = data[i++];
}
if (lookAheadSize == LOOK_AHEAD_SIZE || i == size) {
int matchPos;
int len = searchMatch(0, &matchPos);
if (len >= MIN_MATCH) {
outputCode(matchPos, len);
slideWindow(len);
lookAheadSize -= len;
memmove(lookAheadBuffer, lookAheadBuffer + len, lookAheadSize);
} else {
outputLiteral(lookAheadBuffer[0]);
slideWindow(1);
lookAheadSize--;
memmove(lookAheadBuffer, lookAheadBuffer + 1, lookAheadSize);
}
}
}
}
int main()
{
unsigned char data[] = "hello worldhello worldhello worldhello worldhello world";
compress(data, strlen(data));
return 0;
}
```
代码中 `WINDOW_SIZE` 定义了滑动窗口的大小,`LOOK_AHEAD_SIZE` 定义了查找缓冲区的大小,`MIN_MATCH` 定义了最小匹配长度。
`slideWindow` 函数实现了滑动窗口的功能,将窗口向左移动 `n` 个字节。
`searchMatch` 函数实现了查找匹配的功能,从窗口的起始位置开始查找最长的匹配。
`outputLiteral` 函数输出字面量。
`outputCode` 函数输出匹配码。
`compress` 函数是 LZSS 算法的核心实现,它将输入数据压缩成字面量和匹配码的序列,并输出到标准输出流中。
阅读全文
相关推荐
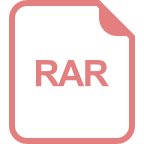
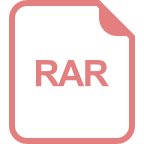















