DS18B20程序设计流程
时间: 2023-12-01 11:04:33 浏览: 59
DS18B20是一种数字温度传感器,以下是DS18B20程序设计的基本流程:
1. 初始化DS18B20传感器:需要设置GPIO引脚的方向和电平状态,以及进行DS18B20特有的初始化过程。
2. 发送指令给DS18B20传感器:发送“读温度”或“写寄存器”等命令,这些命令需要按照一定的时序进行发送。
3. 等待DS18B20传感器响应:在发送完命令后,需要等待DS18B20传感器的响应,以确保传感器已经准备好进行数据传输。
4. 读取DS18B20传感器数据:在准备好的时候,就可以读取DS18B20传感器返回的温度数据,这些数据需要根据DS18B20的协议进行解码和计算。
下面是一个简单的DS18B20程序设计示例,使用STM32F4系列的芯片和Keil MDK进行开发:
```c
#include "stm32f4xx.h"
#include "ds18b20.h"
// 定义DS18B20引脚
#define DS18B20_GPIO_PORT GPIOB
#define DS18B20_GPIO_PIN GPIO_Pin_12
// DS18B20初始化函数
void DS18B20_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
// 使能GPIOB时钟
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOB, ENABLE);
// 配置GPIOB.12为输出模式
GPIO_InitStructure.GPIO_Pin = DS18B20_GPIO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(DS18B20_GPIO_PORT, &GPIO_InitStructure);
}
// 发送DS18B20指令
void DS18B20_SendCommand(uint8_t command)
{
uint8_t i;
// 发送8位数据
for (i = 0; i < 8; i++)
{
// 发送位0或位1
GPIO_SetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
if (command & (1 << i))
{
GPIO_ResetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
}
// 等待一段时间
Delay_us(50);
// 恢复引脚状态
GPIO_SetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
}
}
// 读取DS18B20数据
uint16_t DS18B20_ReadData(void)
{
uint16_t data = 0;
uint8_t i;
// 发送读取温度命令
DS18B20_SendCommand(0xCC); // 跳过ROM指令
DS18B20_SendCommand(0x44); // 温度转换指令
// 等待转换完成
Delay_ms(800);
// 发送读取温度命令
DS18B20_SendCommand(0xCC); // 跳过ROM指令
DS18B20_SendCommand(0xBE); // 读取温度数据指令
// 读取16位数据
for (i = 0; i < 16; i++)
{
// 读取位0或位1
GPIO_SetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
if (GPIO_ReadInputDataBit(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN))
{
data |= (1 << i);
}
// 等待一段时间
Delay_us(50);
}
return data;
}
// 计算DS18B20温度
float DS18B20_GetTemperature(uint16_t data)
{
float temperature = 0;
// 将16位数据转换为10位温度数据
if (data & 0x8000)
{
temperature = (float)(~data + 1) * -0.0625;
}
else
{
temperature = (float)data * 0.0625;
}
return temperature;
}
int main(void)
{
float temperature;
// 初始化DS18B20传感器
DS18B20_Init();
while (1)
{
// 读取温度数据
uint16_t data = DS18B20_ReadData();
// 计算温度值
temperature = DS18B20_GetTemperature(data);
// 在串口终端输出温度值
printf("Temperature: %.1f C\r\n", temperature);
// 延时1秒
Delay_ms(1000);
}
}
```
相关推荐
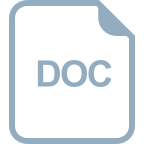
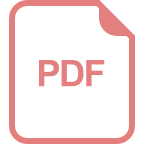
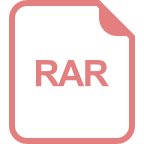














