opencv中直线方程怎么显示
时间: 2024-02-17 08:10:43 浏览: 23
在OpenCV中,一条直线可以由其斜率和截距表示,也可以由两个点的坐标表示。如果你已知直线的斜率和截距,可以使用 `cv::line()` 函数来在图像上绘制出这条直线。示例代码如下:
```cpp
cv::Mat img = cv::imread("test.jpg", cv::IMREAD_GRAYSCALE);
cv::Mat dst = img.clone();
double slope = 1.0; // 直线斜率
double intercept = 0.0; // 直线截距
cv::Point pt1, pt2;
pt1.x = 0;
pt1.y = cvRound(slope * pt1.x + intercept);
pt2.x = dst.cols - 1;
pt2.y = cvRound(slope * pt2.x + intercept);
cv::line(dst, pt1, pt2, cv::Scalar(255, 0, 0), 2);
cv::imshow("original image", img);
cv::imshow("result", dst);
cv::waitKey(0);
```
在上面的示例代码中,我们使用了 `cv::line()` 函数来绘制直线,并使用了 `cvRound()` 函数对结果进行了四舍五入。最后将结果显示出来,可以在图像上看到一条直线。
相关问题
python使用opencv对二值图像进行直线拟合并显示,输出直线方程
以下是使用OpenCV在Python中对二值图像进行直线拟合并显示,并输出直线方程的代码示例:
```python
import cv2
import numpy as np
# 读取二值图像
img = cv2.imread('binary_image.png', cv2.IMREAD_GRAYSCALE)
# 寻找图像中的直线
lines = cv2.HoughLinesP(img, rho=1, theta=np.pi/180, threshold=100, minLineLength=100, maxLineGap=10)
# 在原图上绘制直线
for line in lines:
x1, y1, x2, y2 = line[0]
cv2.line(img, (x1, y1), (x2, y2), (0, 255, 0), 2)
# 输出直线方程
for line in lines:
x1, y1, x2, y2 = line[0]
k = (y2 - y1) / (x2 - x1)
b = y1 - k * x1
print('y = {}x + {}'.format(k, b))
# 显示处理后的图像
cv2.imshow('Result', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个示例中,我们使用了OpenCV的`cv2.HoughLinesP`函数来寻找图像中的直线,并使用`cv2.line`函数在原图上绘制出找到的直线。然后,我们通过直线的两点坐标计算直线的斜率和截距,输出直线方程。最后,使用`cv2.imshow`函数显示处理后的图像。
Java opencv HoughLinesP 线性回归拟合直线
使用OpenCV中的HoughLinesP函数进行线性回归拟合直线的步骤如下:
1. 导入JavaCV和OpenCV库。
2. 读取图像并转换为灰度图像。
3. 对图像进行Canny边缘检测。
4. 使用HoughLinesP函数检测直线。
5. 对检测到的直线进行线性回归拟合,得到最佳拟合直线。
下面是完整的Java代码示例:
```java
import org.bytedeco.javacpp.opencv_core.*;
import org.bytedeco.javacpp.opencv_imgproc.*;
import org.bytedeco.javacpp.opencv_highgui.*;
import org.bytedeco.javacpp.*;
public class HoughLinesPExample {
public static void main(String[] args) {
// 读取图像
Mat image = imread("test.jpg");
// 转换为灰度图像
Mat grayImage = new Mat();
cvtColor(image, grayImage, COLOR_BGR2GRAY);
// Canny边缘检测
Mat edges = new Mat();
Canny(grayImage, edges, 50, 200, 3, false);
// HoughLinesP函数检测直线
Mat lines = new Mat();
HoughLinesP(edges, lines, 1, Math.PI / 180, 50, 50, 10);
// 计算直线斜率和截距的平均值
double slopeSum = 0, interceptSum = 0;
for (int i = 0; i < lines.rows(); i++) {
double[] data = lines.get(i, 0);
double x1 = data[0], y1 = data[1], x2 = data[2], y2 = data[3];
double slope = (y2 - y1) / (x2 - x1);
double intercept = y1 - slope * x1;
slopeSum += slope;
interceptSum += intercept;
}
double slopeAvg = slopeSum / lines.rows();
double interceptAvg = interceptSum / lines.rows();
// 绘制拟合直线
Point pt1 = new Point(0, interceptAvg);
Point pt2 = new Point(image.cols(), slopeAvg * image.cols() + interceptAvg);
line(image, pt1, pt2, new Scalar(0, 0, 255), 2, LINE_AA, 0);
// 显示图像
imshow("HoughLinesP Example", image);
waitKey();
}
}
```
在上面的代码中,我们使用了OpenCV中的HoughLinesP函数来检测直线。该函数的参数解释如下:
- edges:输入的边缘图像。
- lines:输出的直线向量。
- rho:距离分辨率。
- theta:角度分辨率。
- threshold:直线被检测出来的最小投票数。
- minLineLength:直线的最小长度。
- maxLineGap:直线上的点与点之间最大允许的间隔。
通过计算检测到的直线的斜率和截距的平均值,我们可以得到最佳拟合直线的方程。最后,我们使用line函数绘制出拟合直线,并使用imshow函数显示图像。
相关推荐
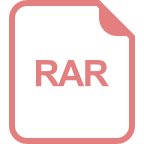












