python直线检测计算交点_Python+OpenCV图像处理——实现直线检测
时间: 2023-11-21 21:04:53 浏览: 341
在Python和OpenCV中实现直线检测,可以使用Hough变换来检测直线。Hough变换是一种常用的图像处理方法,可用于检测直线、圆等几何形状。
以下是一个简单的示例代码,使用Hough变换来检测直线并计算交点:
```python
import cv2
import numpy as np
# 读取图像
img = cv2.imread('test.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 边缘检测
edges = cv2.Canny(gray, 50, 150, apertureSize=3)
# Hough变换检测直线
lines = cv2.HoughLines(edges, 1, np.pi/180, 200)
# 计算交点
points = []
for i in range(len(lines)):
for j in range(i+1, len(lines)):
rho1, theta1 = lines[i][0]
rho2, theta2 = lines[j][0]
if abs(theta1 - theta2) < np.pi/4:
continue
a = np.array([[np.cos(theta1), np.sin(theta1)], [np.cos(theta2), np.sin(theta2)]])
b = np.array([rho1, rho2])
x0, y0 = np.linalg.solve(a, b)
points.append((int(x0), int(y0)))
# 绘制直线和交点
for line in lines:
rho, theta = line[0]
a = np.cos(theta)
b = np.sin(theta)
x0 = a*rho
y0 = b*rho
x1 = int(x0 + 1000*(-b))
y1 = int(y0 + 1000*(a))
x2 = int(x0 - 1000*(-b))
y2 = int(y0 - 1000*(a))
cv2.line(img, (x1,y1), (x2,y2), (0,0,255), 2)
for point in points:
cv2.circle(img, point, 5, (0,255,0), -1)
# 显示图像
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在代码中,首先读取图像并进行灰度转换和边缘检测。然后使用Hough变换检测直线,并计算交点。最后绘制直线和交点,并显示图像。
需要注意的是,在计算交点时,需要将两条直线的极坐标表示转换为直角坐标表示,并使用线性方程组求解。
希望这个例子能够帮助到你实现直线检测并计算交点。
阅读全文
相关推荐
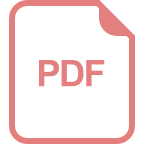
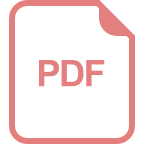

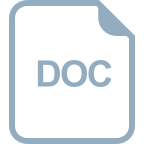
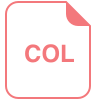
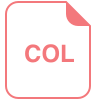
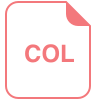
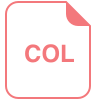
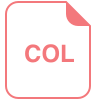
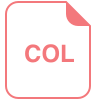







