写一段python程序,从file.ini配置文件里读取一系列key的value值,作为一个记录写入MySQL
时间: 2023-09-11 12:05:03 浏览: 150
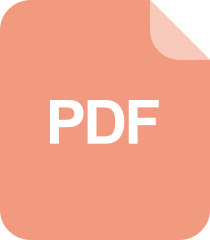
python读取配置文件方式(ini、yaml、xml)
### 回答1:
```python
import configparser
import mysql.connector
# 读取配置文件
config = configparser.ConfigParser()
config.read('file.ini')
# 连接MySQL数据库
conn = mysql.connector.connect(
host=config['MYSQL']['host'],
user=config['MYSQL']['user'],
password=config['MYSQL']['password'],
database=config['MYSQL']['database']
)
# 使用cursor()方法获取操作游标
cursor = conn.cursor()
# 循环读取配置文件中的key和value
for key, value in config['VALUES'].items():
# 执行SQL语句,将记录插入到MySQL中
cursor.execute("INSERT INTO records (key, value) VALUES (%s, %s)", (key, value))
# 提交事务
conn.commit()
# 关闭数据库连接
cursor.close()
conn.close()
```
需要注意的是,这段程序需要MySQL Connector Python库的支持,如果没有安装,需要先运行`pip install mysql-connector-python`来安装。
配置文件 file.ini 需要提前创建,参考格式如下
```
[MYSQL]
host = localhost
user = root
password = root
database = test
[VALUES]
key1 = value1
key2 = value2
key3 = value3
```
这段程序的主要思路是读取配置文件中的key和value,然后将它们作为记录插入到MySQL中。
### 回答2:
可以使用`configparser`模块读取配置文件,并使用`mysql-connector-python`模块将数据写入MySQL数据库。
首先,确保你已经安装了`configparser`和`mysql-connector-python`模块,你可以使用以下指令进行安装:
```python
pip install configparser mysql-connector-python
```
接下来,可以按照以下代码编写一个Python程序:
```python
import configparser
import mysql.connector
# 读取配置文件
config = configparser.ConfigParser()
config.read('file.ini')
# 设置数据库连接参数
db_config = {
'user': config.get('Database', 'user'),
'password': config.get('Database', 'password'),
'host': config.get('Database', 'host'),
'database': config.get('Database', 'database')
}
# 建立数据库连接
cnx = mysql.connector.connect(**db_config)
cursor = cnx.cursor()
# 从配置文件读取需要写入的key和value值
keys = config.get('Data', 'keys').split(',')
values = config.get('Data', 'values').split(',')
# 构建MySQL查询语句
query = "INSERT INTO your_table (key_col, value_col) VALUES (%s, %s)"
# 执行插入操作
for key, value in zip(keys, values):
cursor.execute(query, (key, value))
# 提交更改
cnx.commit()
# 关闭连接
cursor.close()
cnx.close()
```
上面的代码假设配置文件`file.ini`的格式如下:
```
[Database]
user = your_username
password = your_password
host = your_host
database = your_database
[Data]
keys = key1,key2,key3
values = value1,value2,value3
```
请将代码中的`your_table`替换为你的MySQL表名,`key_col`和`value_col`替换为你的表中对应的列名。
这样,程序会读取`file.ini`配置文件中`Data`节下的`keys`和`values`的值,并将其写入MySQL数据库。
### 回答3:
下面是一个使用Python从file.ini配置文件中读取一系列key的value值,并将其作为记录写入MySQL数据库的示例程序:
```python
import configparser
import mysql.connector
# 读取配置文件
config = configparser.ConfigParser()
config.read('file.ini')
# 获取数据库连接信息
host = config.get('Database', 'host')
user = config.get('Database', 'user')
password = config.get('Database', 'password')
database = config.get('Database', 'database')
# 连接到MySQL数据库
conn = mysql.connector.connect(
host=host,
user=user,
password=password,
database=database
)
cursor = conn.cursor()
# 读取并处理配置文件中的key-value数据,插入数据库
for key in config.options('Data'):
value = config.get('Data', key)
# 构建插入语句
sql = "INSERT INTO table_name (key, value) VALUES (%s, %s)"
data = (key, value)
# 执行插入操作
cursor.execute(sql, data)
conn.commit()
# 关闭数据库连接
cursor.close()
conn.close()
```
在该示例中,我们使用`configparser`模块来读取配置文件`file.ini`的内容。假设配置文件如下所示:
```
[Database]
host = localhost
user = username
password = password
database = dbname
[Data]
key1 = value1
key2 = value2
key3 = value3
...
```
我们首先获取数据库连接信息,然后连接到MySQL数据库。接下来,对于配置文件中`[Data]`部分的每个key-value对,我们使用SQL插入语句将其插入到一个名为`table_name`的表中。最后,我们关闭数据库连接。
请注意,你需要将程序中的`host`,`user`,`password`,`database`和`table_name`替换为实际的数据库信息和表名。
阅读全文
相关推荐
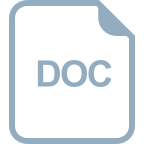
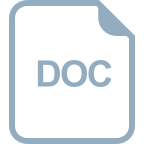

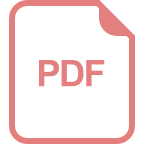
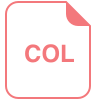
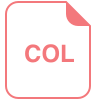
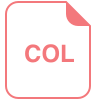
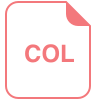
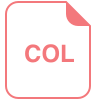
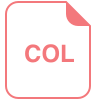
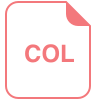
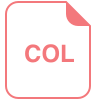
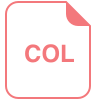
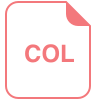
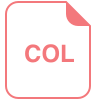
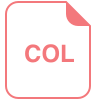
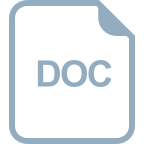
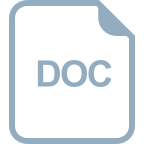