Python读写大数据集:MySQL性能优化的最佳实践
发布时间: 2024-09-12 03:53:54 阅读量: 104 订阅数: 76 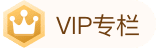
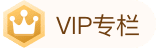
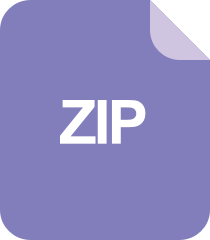
果壳处理器研究小组(Topic基于RISCV64果核处理器的卷积神经网络加速器研究)详细文档+全部资料+优秀项目+源码.zip
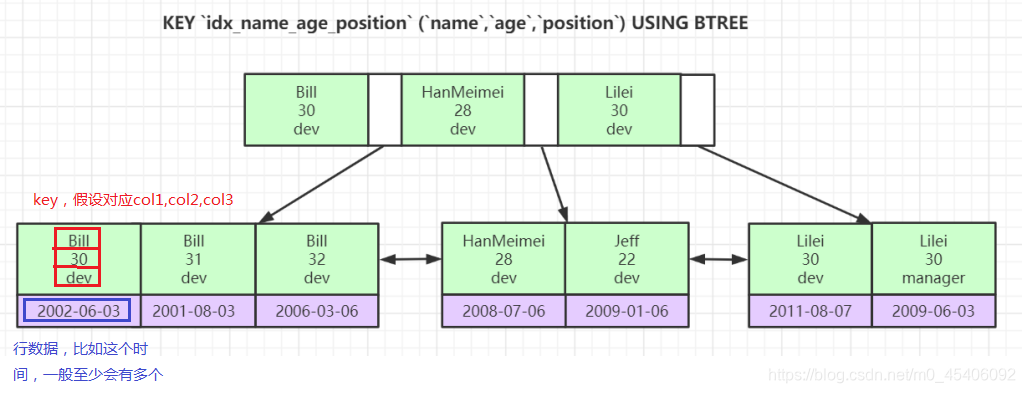
# 1. Python与MySQL交互基础
在现代数据驱动的应用程序中,Python与MySQL的交互是经常遇到的需求。Python拥有简洁的语法和强大的第三方库支持,其中`mysql-connector-python`和`pymysql`是广泛使用的库,它们允许Python程序连接到MySQL数据库并进行数据操作。交互过程通常包括连接数据库、执行SQL查询语句、处理结果集以及关闭数据库连接。
## 连接MySQL数据库
首先,需要安装`mysql-connector-python`库:
```bash
pip install mysql-connector-python
```
然后,可以通过以下代码连接到MySQL数据库:
```python
import mysql.connector
# 建立连接
connection = mysql.connector.connect(
user='yourusername',
password='yourpassword',
host='***.*.*.*',
database='mydatabase'
)
# 创建游标对象
cursor = connection.cursor()
# 执行SQL语句
cursor.execute("SELECT * FROM mytable")
# 获取查询结果
results = cursor.fetchall()
# 输出结果
for row in results:
print(row)
# 关闭游标和连接
cursor.close()
connection.close()
```
在上述代码中,我们完成了数据库连接、创建游标、执行查询、处理结果以及关闭连接的全过程。实际应用中,这段代码会根据具体的需求有所变化,例如,根据参数化查询的需求,可以使用`cursor.execute()`方法执行参数化的SQL语句。
通过Python与MySQL的交互基础,我们可以构建更复杂的数据操作逻辑,为构建高级应用程序奠定基础。在接下来的章节中,我们将深入探讨如何在大数据场景下,实现高效的数据读取与写入,以及如何进行性能优化。
# 2. 大数据集的高效读取技术
大数据的兴起使得开发者在处理数据时面临的挑战越来越大,高效地从数据库中读取大量数据成为了迫切的需求。本章将深入探讨如何使用Python高效地读取大数据集,包括数据库连接池的使用、读取策略的选择以及数据流的处理和转换。
## 2.1 数据库连接池的使用
数据库连接池是一种用于管理数据库连接的技术,它可以显著提高大量数据库操作的性能。在面对大量并发请求时,连接池可以避免频繁地建立和关闭数据库连接,从而减少系统开销。
### 2.1.1 连接池的基本概念
连接池是一种池化资源管理技术,它预先创建一定数量的数据库连接,并将这些连接保存在一个池中。当应用程序需要使用数据库连接时,可以从池中取出一个连接使用,使用完毕后,将连接返回给连接池而不是直接关闭。连接池通过复用连接来提高应用程序的性能,并且能够控制数据库连接的数量,防止资源过度消耗。
### 2.1.2 实现连接池的Python库选择
在Python中,有几个库可以用来实现连接池。比较著名的包括`pymysql`和`psycopg2`,它们分别提供了对MySQL和PostgreSQL数据库的连接池支持。此外,还有一个通用的库`SQLAlchemy`,它支持多种数据库系统,并内置了连接池的功能。为了实现更高级的连接池功能,还可以使用`DBUtils`库,它提供了一个`PooledDB`模块,能够创建连接池并进行高级管理。
### 2.1.3 连接池的配置与性能测试
连接池的配置通常包括最小连接数、最大连接数、连接的获取和回收策略等。以`PooledDB`为例,可以通过设置`mincached`(最小缓存连接数)和`maxcached`(最大缓存连接数)参数来控制连接池的大小。性能测试是验证连接池配置是否合理的重要环节。可以使用`Apache JMeter`或`locust`等工具模拟高并发场景,观察连接池的表现和数据库连接的使用情况。
```python
from PooledDB import PooledDB
import pymysql
# 创建连接池
connection_pool = PooledDB(
creator=pymysql, # 使用 PyMySQL 创建连接
mincached=2, # 最小缓存连接数
maxcached=5, # 最大缓存连接数
maxshared=10, # 最大共享连接数
setsession=['SET NAMES utf8'], # 初始化连接时的命令列表
ping=0 # 连接测试命令,0为不测试
)
# 使用连接池中的连接
connection = connection_pool.connection()
cursor = connection.cursor()
# 执行数据库操作...
cursor.close()
connection.close()
```
在上述代码中,我们创建了一个连接池,并通过连接池获取连接并执行了数据库操作。在性能测试时,我们通过监控连接池中连接的使用情况,以及数据库的响应时间来评估连接池的效果。
## 2.2 读取大数据集的策略
从数据库中读取大数据集时,应考虑策略以优化性能。这一节将介绍分页查询技术、利用游标的高效数据处理以及多线程和异步IO读取技巧。
### 2.2.1 分页查询技术
当数据库中的数据量非常大时,一次性查询出所有数据往往会导致查询时间过长,甚至服务器无响应。分页查询技术通过分批次处理查询结果集,来减少单次查询的负载,提高性能。
```python
cursor.execute("SELECT * FROM big_table LIMIT %s OFFSET %s", (page_size, page * page_size))
```
上述代码展示了如何使用MySQL的`LIMIT`和`OFFSET`子句进行分页查询。在这里,`page_size`是每次查询的数据量,`page`是当前页数。通过逐步增加`page`的值来查询下一页的数据,可以有效地从数据库中读取大量数据而不会对数据库性能造成太大压力。
### 2.2.2 利用游标的高效数据处理
当需要处理的数据量非常大,无法一次性加载到内存中时,可以使用游标进行数据的逐行处理。Python的DB-API提供了游标对象,允许逐行迭代结果集。
```python
with connection.cursor() as cursor:
cursor.execute("SELECT * FROM big_table")
for row in cursor:
process(row)
```
在使用游标时,数据库仅返回一行数据,直到下一行数据被请求。这种方法对内存的需求较低,适合处理大结果集。`process(row)`是处理每行数据的函数,可以根据实际业务需求进行编写。
### 2.2.3 多线程和异步IO读取技巧
对于IO密集型的应用程序,多线程和异步IO是提高性能的有效手段。Python的`threading`模块和`asyncio`库可以用来实现并发读取。
```python
import threading
import pymysql
def fetch_data(page_size, page):
connection = pymysql.connect()
cursor = connection.cursor()
cursor.execute("SELECT * FROM big_table LIMIT %s OFFSET %s", (page_size, page * page_size))
# 处理数据...
connection.close()
threads = []
for i in range(num_pages):
page_size = 100 # 每页数据量
t = threading.Thread(target=fetch_data, args=(page_size, i))
threads.append(t)
t.start()
for t in threads:
t.join()
```
此代码段创建了多个线程,每个线程负责从数据库中获取一定范围的数据。为了防止一次性开启过多线程导致资源竞争,可以使用线程池管理线程的创建和销毁。
对于异步IO,可以使用`aiomysql`库来实现:
```python
import asyncio
import aiomysql
async def fetch_data(page_size, page):
async with aiomysql.create_pool(host='localhost', port=3306, user='user',
password='password', db='db', minsize=1, maxsize=10) as pool:
async with pool.acquire() as conn:
async with conn.cursor() as cursor:
await cursor.execute("SELECT * FROM big_table LIMIT %s OFFSET %s", (page_size, page * page_size))
result = await cursor.fetchall()
# 处理数据...
return result
async def main():
tasks = []
num_pages = 100
page_size = 100
for i in range(num_pages):
tasks.append(fetch_data(page_size, i))
results = await asyncio.gather(*tasks)
# 组合所有结果...
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
loop.close()
```
这里使用了`asyncio`库和`aiomysql`异步驱动来实现异步数据库
0
0
相关推荐
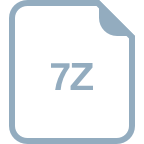
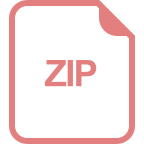
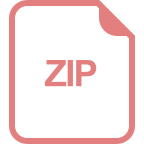
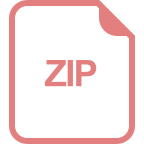
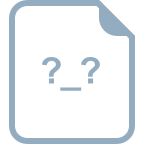
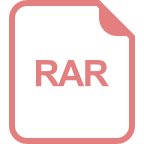
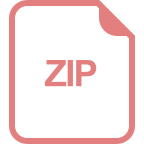