Python中MySQL的数据完整性约束和索引优化:提升数据库性能的关键
发布时间: 2024-09-12 04:35:23 阅读量: 124 订阅数: 76 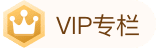
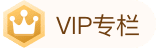
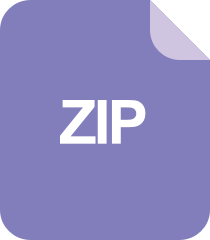
果壳处理器研究小组(Topic基于RISCV64果核处理器的卷积神经网络加速器研究)详细文档+全部资料+优秀项目+源码.zip
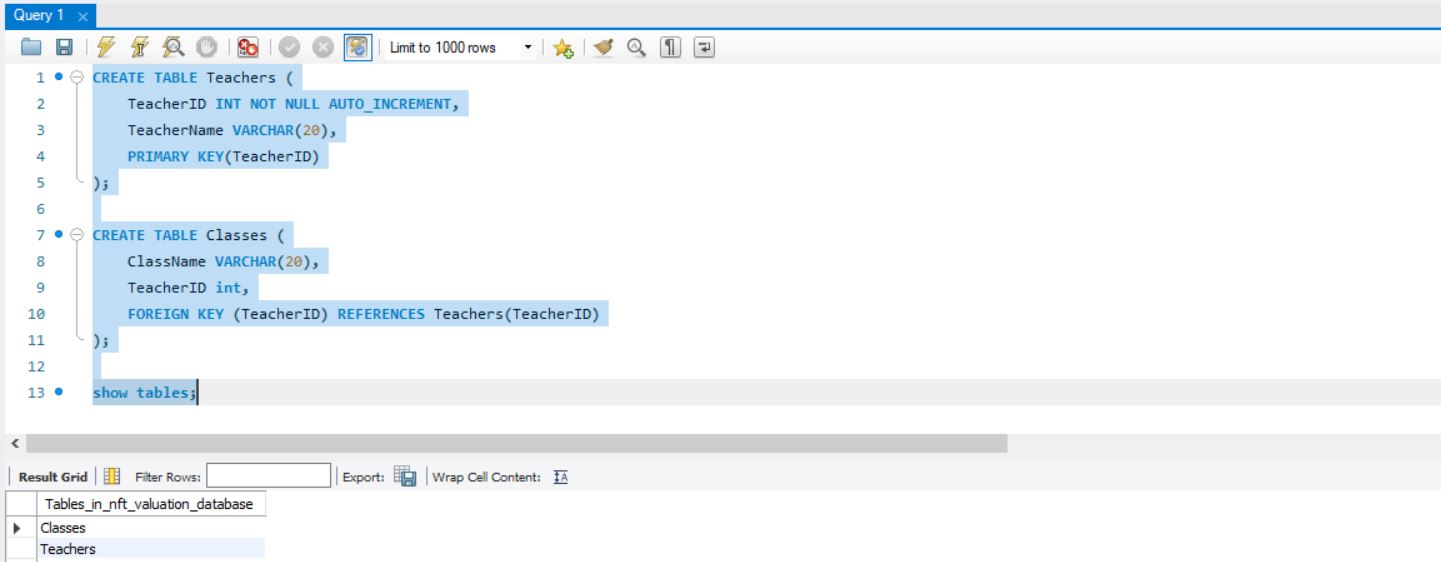
# 1. 数据完整性约束的概念和作用
数据完整性约束是数据库管理系统中确保数据准确性和可靠性的基本机制。其作用在于确保数据库中数据的准确性和一致性,防止无效或者错误的数据输入到数据库中。数据完整性约束的缺失可能会导致数据丢失、数据错误或者数据冗余,从而对数据库系统的正常运行造成影响。
在数据管理的实际工作中,数据完整性约束主要分为三类:实体完整性约束、域完整性约束和参照完整性约束。实体完整性约束确保了数据库中每个实体的唯一性;域完整性约束保证了数据值域的正确性;参照完整性约束则是维护了数据表之间的引用关系,保证了数据的一致性。
对于企业而言,有效地利用数据完整性约束可以大幅提高数据处理的准确度和效率,减少数据清洗和维护的成本。而在数据挖掘和分析的过程中,数据完整性约束也是保证数据质量,确保分析结果可信度的重要环节。因此,深入理解并正确应用数据完整性约束对于构建高效、稳定、可信赖的数据库系统至关重要。
# 2. 在Python中实现MySQL的数据完整性约束
## 2.1 理解数据完整性约束的类型
数据完整性约束是数据库管理系统用来确保数据的准确性和有效性的规则。它们对于保护数据免受意外或恶意的改动至关重要。在关系数据库中,数据完整性约束分为几类,每一类针对数据库的不同方面提供保护。
### 2.1.1 实体完整性约束
实体完整性约束确保表中的每一行都是唯一的。这是通过主键约束实现的,主键是表中的一列或多列,其值唯一标识表中的每一行。主键约束还有助于强制参照完整性,确保外键引用总是对应于有效的主键值。
### 2.1.2 域完整性约束
域完整性约束规定了某一列中允许的数据类型、格式以及值的范围。这类约束确保列中的数据总是符合预期的数据类型,如整数、浮点数、字符串、日期等。还可以进一步限制数据的格式,如电子邮件地址或电话号码的格式。
### 2.1.3 参照完整性约束
参照完整性约束确保表之间的数据引用关系的正确性。这通常通过外键约束来实现,它要求一个表中的特定列(外键)的值必须匹配另一个表中的主键值,或者该列允许为NULL(如果参照的主键不存在)。参照完整性有助于维护数据库的一致性和整体数据质量。
## 2.2 在Python中使用数据完整性约束
Python通过多种方式与MySQL数据库交互,允许开发者在应用程序中实现数据完整性约束。我们来看看一些最常见的方法。
### 2.2.1 Django框架中的数据完整性实现
Django是一个高级的Python Web框架,它鼓励快速开发和干净、实用的设计。Django的模型层提供了一种简单而强大的方式来定义和操作数据库中的数据。
```python
from django.db import models
class Publisher(models.Model):
name = models.CharField(max_length=300)
address = models.CharField(max_length=500)
website = models.URLField()
class Book(models.Model):
title = models.CharField(max_length=300)
publisher = models.ForeignKey(Publisher, on_delete=models.CASCADE)
# 其他字段...
```
在上面的例子中,`ForeignKey` 表示参照完整性约束,它确保了所有书籍都有一个有效的出版商。
### 2.2.2 SQLAlchemy ORM的约束应用
SQLAlchemy是Python中一个流行的数据库工具包和对象关系映射(ORM)库,它提供了丰富的接口来定义数据完整性约束。
```python
from sqlalchemy import create_engine, Column, Integer, String, ForeignKey
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import relationship
Base = declarative_base()
class Publisher(Base):
__tablename__ = 'publisher'
id = Column(Integer, primary_key=True)
name = Column(String)
address = Column(String)
website = Column(String)
class Book(Base):
__tablename__ = 'book'
id = Column(Integer, primary_key=True)
title = Column(String)
publisher_id = Column(Integer, ForeignKey('publisher.id'))
publisher = relationship('Publisher', back_populates='books')
Publisher.books = relationship('Book', order_by=Book.id)
```
在这个例子中,`ForeignKey` 同样表示参照完整性约束,它表明书籍和出版商之间的一对多关系。
### 2.2.3 手动使用SQL语句设置约束
当使用原生Python连接到MySQL时,可以直接通过SQL语句来定义约束。
```python
import mysql.connector
from mysql.connector import Error
try:
connection = mysql.connector.connect(
host='localhost',
database='test_db',
user='user',
password='password'
)
cursor = connection.cursor()
cursor.execute("""
CREATE TABLE Publisher (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(300) NOT NULL,
address VARCHAR(500) NOT NULL,
website VARCHAR(255)
);
CREATE TABLE Book (
id INT AUTO_INCREMENT PRIMARY KEY,
title VARCHAR(300) NOT NULL,
publisher_id INT,
FOREIGN KEY (publisher_id) REFERENCES Publisher(id)
);
""")
except Error as e:
print(f"Error: {e}")
finally:
if connection.is_connected():
cursor.close()
connection.close()
```
此代码段创建了两个表,并在它们之间建立了参照完整性约束。`FOREIGN KEY` 用于确保`publisher_id`在`Book`表中引用`Publisher`表中的现有记录。
## 2.3 数据完整性约束的实践案例分析
了解了数据完整性约束的类型和在Python中的使用方法后,接下来将通过一些实践案例来加深理解。
### 2.3.1 数据库设计阶段的约束应用
在数据库设计阶段,应用数据完整性约束可以确保数据在输入时就遵循预设的规则,从而减少后续的数据清洗和校验工作。
### 2.3.2 数据操作时的约束校验实例
在数据操作时(如插入、更新或删除数据),约束校验机制将自动执行,以确保数据的准确性。任何违反这些规则的操作都会被数据库管理系统拒绝,并可能返回错误信息给用户。
接下来,我们将详细探讨索引优化的基本原理与实践,以及数据完整性约束和索引优化的高级应用,为数据库系统性能的提升提供更全面的视角。
# 3. 索引优化的基本原理与实践
## 3.1 索引优化的重要性
索引优化对于数据库的性能至关重要。通过索引,数据库系统可以快速定位到数据所在的位置,显著减少数据搜索的时间。理解索引的工作原理和它们对查询性能的影响,是进行有效数据库管理的基础。
### 3.1.1 索引的工作原理
索引类似于书籍的目录,它能够帮助数据库快速定位到数据记录,而不必扫描整个数据表。在物理层面上,索引通常是一棵B-Tree结构,通过在每个索引节点上维护键值和指针,实现了快速查找功能。索引可以是单一列的,也可以是多个列的组合。
```sql
CREATE INDEX idx_name ON table_name (column_name);
```
在创建索引后,数据库会自动维护索引的更新,以保证数据的一致性。索引的查询操作通常非常快速,因为它们极大地减少了需要检查的数据量。
### 3.1.2 索引对
0
0
相关推荐
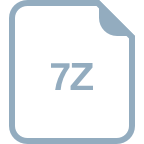
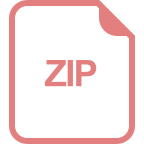
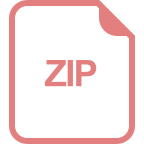
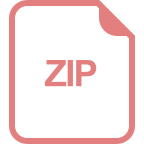
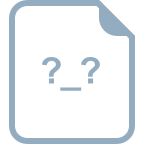
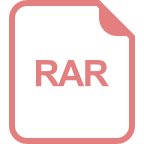
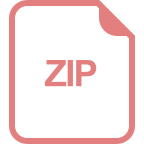