定义一个Computer类,有属性public int state 表示状态码。有2个方法,一个是run()方法。当state 为1时,在方法里显示“电脑成功运行”和“老师开始上课了”;当state 为2时,在方法里抛出自定义异常LanPingException,显示"电脑蓝屏了"。当state 为3时,在在方法里抛出自定义异常MaoYanException,显示"电脑冒烟了"。还有一个recst()方法,可重启电脑,打印“电脑重启中…..”和“老师开始上课”。 定义一个Teacher类,用Random随机生成(1-3的范围)state状态码,然后运行电脑,并进行异常处理。如果电脑蓝屏了,就重启电脑。如果电脑冒烟了,就抛出自定义异常NoPlanException显示“上课无法继续,需要修理电脑”。
时间: 2024-03-25 16:38:15 浏览: 137
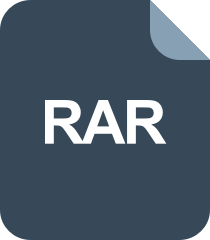
计算机类.
好的,以下是代码实现:
```java
//定义自定义异常LanPingException
class LanPingException extends Exception {
public LanPingException(String message) {
super(message);
}
}
//定义自定义异常MaoYanException
class MaoYanException extends Exception {
public MaoYanException(String message) {
super(message);
}
}
//定义Computer类
class Computer {
public int state;
public void run() throws LanPingException, MaoYanException {
if (state == 1) {
System.out.println("电脑成功运行");
System.out.println("老师开始上课了");
} else if (state == 2) {
throw new LanPingException("电脑蓝屏了");
} else if (state == 3) {
throw new MaoYanException("电脑冒烟了");
}
}
public void recst() {
System.out.println("电脑重启中.....");
System.out.println("老师开始上课");
}
}
//定义Teacher类
class Teacher {
public static void main(String[] args) {
Computer computer = new Computer();
Random random = new Random();
computer.state = random.nextInt(3) + 1;
try {
computer.run();
} catch (LanPingException e) {
System.out.println(e.getMessage());
computer.recst();
} catch (MaoYanException e) {
System.out.println(e.getMessage());
throw new RuntimeException("上课无法继续,需要修理电脑");
}
}
}
```
在Teacher类中,我们使用了Random类随机生成了1-3的状态码,然后调用Computer类的run()方法进行异常处理。如果出现了LanPingException异常,就重启电脑,并打印错误信息;如果出现了MaoYanException异常,就抛出自定义异常NoPlanException并打印错误信息。
阅读全文
相关推荐
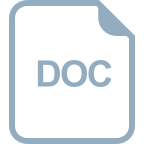

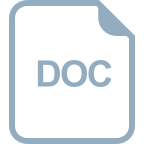
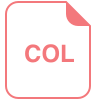
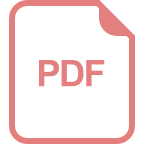
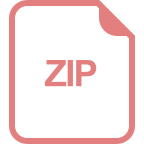
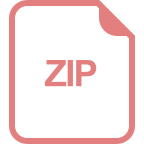
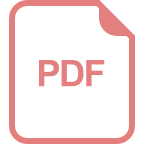
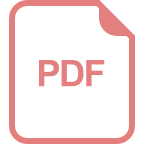
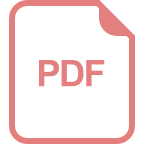