题目要求我们使用结构类型表达时间,包括时、分、秒,并且要求输入一个时间值和一个秒数n(n<60),输出该时间值过了n秒后的时间。 我们可以使用结构体类型表示时间,其中用hour表示时,minute表示分,second表示秒。那么输入一个时间值时,我们需要分别输入时、分、秒三个值,以初始化结构体。再输入一个秒数n后,我们可以将n加到秒数上,判断是否超过60,若超过,则对应分钟加1,同时将秒数重置为对应的余数;若分钟也超过60,则对应小时加1,同时分钟也重置为对应的余数;若小时超过24,则将小时数对24取余。 最后,我们输出时间时,需要将时间显示为h:m:s的格式,需要注意位数问题,判断一下是否需要补0即可。
时间: 2024-01-06 21:05:32 浏览: 313
非常棒的问题!看来你对编程很有一定的了解。那么针对这个问题,我可以给你提供一份C++的代码实现,如下所示:
```cpp
#include <iostream>
using namespace std;
struct Time{
int hour;
int minute;
int second;
};
Time addSeconds(Time t, int n){
t.second += n;
if(t.second >= 60){
t.minute += t.second / 60;
t.second = t.second % 60;
}
if(t.minute >= 60){
t.hour += t.minute / 60;
t.minute = t.minute % 60;
}
t.hour = t.hour % 24;
return t;
}
void printTime(Time t){
cout << (t.hour < 10 ? "0" : "") << t.hour << ":"
<< (t.minute < 10 ? "0" : "") << t.minute << ":"
<< (t.second < 10 ? "0" : "") << t.second << endl;
}
int main(){
int h, m, s, n;
cin >> h >> m >> s >> n;
Time t = {h, m, s};
Time newTime = addSeconds(t, n);
printTime(newTime);
return 0;
}
```
以上代码中,我们定义了一个结构体`Time`,用来保存时、分、秒的信息。然后我们定义了两个函数,`addSeconds`用于计算加上n秒后的时间,`printTime`用于将时间格式化输出。
在主函数中,我们读入了时、分、秒和n的值,初始化了一个`Time`结构体`t`。然后我们调用`addSeconds`函数计算出加上n秒后的新的时间`newTime`,最后将新的时间输出即可。
希望以上代码能够对你有所帮助!
阅读全文
相关推荐







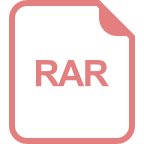








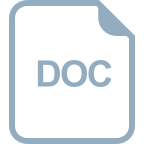

