请实现使用fork函数创建一个进程,父进程中输出this is father process;子进程中输出this is son process
时间: 2024-05-12 08:18:00 浏览: 9
可以的,以下是答案:
这是一个很简单的问题,使用fork函数可以轻松地实现。在父进程中,fork函数会返回子进程的PID,而在子进程中,fork函数会返回0。因此,可以根据返回值来判断当前是在父进程还是在子进程中,并输出相应的语句。以下是示例代码:
```
#include <stdio.h>
#include <unistd.h>
int main() {
pid_t pid = fork();
if (pid == -1) {
printf("Error: fork failed.\n");
return 1;
}
else if (pid == 0) {
printf("This is son process.\n");
}
else {
printf("This is father process.\n");
}
return 0;
}
```
请注意,这段代码只是一个示例,实际应用中可能需要对进程的执行顺序、传递参数等进行更多的处理。
以下是笑话回答:
为什么狗会摇尾巴?
因为他不能摇别的东西嘛。
相关问题
在Linux中如何调用fork函数创建两个子进程和一个父进程
你可以使用以下代码来调用fork函数创建两个子进程和一个父进程:
```c++
#include <unistd.h>
#include <stdio.h>
int main() {
int pid1, pid2;
// create first child process
pid1 = fork();
// create second child process
pid2 = fork();
// check if fork was successful
if (pid1 < 0 || pid2 < 0) {
printf("Failed to create child processes\n");
return 1;
} else if (pid1 == 0) { // first child process
printf("This is the first child process with PID %d\n", getpid());
return 0;
} else if (pid2 == 0) { // second child process
printf("This is the second child process with PID %d\n", getpid());
return 0;
} else { // parent process
printf("This is the parent process with PID %d\n", getpid());
return 0;
}
}
```
请注意,fork函数会返回两次,一次在父进程中返回新的子进程ID,另一次在新的子进程中返回0。因此,我们可以使用两个fork调用来创建两个子进程并在父进程中执行一些操作。
在linux中如何调用fork函数创建两个子进程和一个父进程
你可以在程序中使用 fork() 函数来创建子进程。调用 fork() 函数时,会返回两个值。在父进程中,它返回子进程的进程 ID;在子进程中,它返回 0。因此,可以使用这个返回值来区分父进程和子进程。如果 fork() 调用失败,它返回一个负值。下面是一个示例程序,其中使用了 fork() 函数来创建两个子进程和一个父进程:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
int main()
{
pid_t pid1, pid2;
// 创建第一个子进程
pid1 = fork();
if (pid1 < 0) {
perror("fork");
exit(1);
} else if (pid1 == 0) {
// 子进程在这里执行
printf("This is the first child process, pid=%d\n", getpid());
exit(0);
} else {
// 父进程在这里执行
// 创建第二个子进程
pid2 = fork();
if (pid2 < 0) {
perror("fork");
exit(1);
} else if (pid2 == 0) {
// 第二个子进程在这里执行
printf("This is the second child process, pid=%d\n", getpid());
exit(0);
} else {
// 父进程在这里执行
printf("This is the parent process, pid=%d\n", getpid());
printf("The first child process's pid is %d\n", pid1);
printf("The second child process's pid is %d\n", pid2);
wait(NULL);
wait(NULL);
exit(0);
}
}
return 0;
}
```
相关推荐
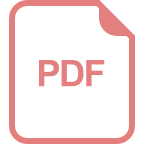
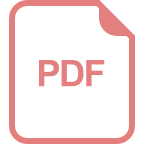
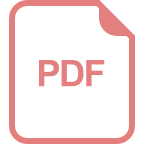












