用汇编语言对存储器中的一组双字进行排序,并将结果显示在屏幕上,并采用基址+变址+偏移的寻址方式
时间: 2023-11-28 08:51:30 浏览: 150
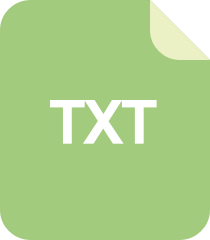
汇编语言排序序程序并显示
以下是用汇编语言对存储器中的一组双字进行排序,并将结果显示在屏幕上,并采用基址+变址+偏移的寻址方式的代码:
```
; Sort and display a set of double words in memory using base+index+offset addressing
section .data
prompt db "Enter the number of double words to sort: ", 0
input_format db "%d", 0
output_format db "%d ", 0
newline db 10, 0
array_size dd 0
array dd 0
section .text
global _start
_start:
; Prompt user for number of elements to sort
mov eax, 4
mov ebx, 1
mov ecx, prompt
mov edx, len(prompt)
int 0x80
; Read user input for array size
mov eax, 3
mov ebx, 0
mov ecx, array_size
mov edx, 4
int 0x80
; Allocate memory for array
shl dword [array_size], 2 ; multiply by 4 to get number of bytes needed
mov eax, 45 ; system call for brk (allocate memory)
mov ebx, 0
mov ecx, array_size
int 0x80
mov [array], eax ; save memory address of array
; Read user input for array elements
mov esi, [array] ; set index register to point to start of array
mov ecx, [array_size]
mov edx, input_format
read_loop:
cmp ecx, 0
je sort_array
push ecx
push edx
push esi
mov eax, 3
mov ebx, 0
mov ecx, esi
mov edx, 4
int 0x80
add esi, 4 ; increment index register by 4 to point to next element
pop esi
pop edx
pop ecx
dec ecx
jmp read_loop
; Sort array using bubble sort algorithm
sort_array:
mov ebx, [array_size]
mov esi, [array]
mov ecx, ebx
dec ecx
outer_loop:
cmp ecx, 0
je print_array
mov edx, 0
inner_loop:
cmp edx, ecx
je outer_loop
mov eax, [esi+edx*4] ; load current element
cmp eax, [esi+(edx+1)*4] ; compare with next element
jle skip_swap
; swap elements if current element is greater than next element
xchg eax, [esi+(edx+1)*4]
mov [esi+edx*4], eax
skip_swap:
inc edx
jmp inner_loop
; Print sorted array to screen
print_array:
mov eax, 4
mov ebx, 1
mov ecx, [array]
mov edx, [array_size]
mov esi, output_format
print_loop:
push edx
push ecx
push esi
int 0x80
add esi, 2 ; increment output format string by 2 to skip over "%d" format specifier
add ecx, 4 ; increment index register by 4 to point to next element
pop esi
pop ecx
pop edx
loop print_loop
; Exit program
mov eax, 1
xor ebx, ebx
int 0x80
len equ $-prompt
```
这段代码使用了基址+变址+偏移的寻址方式来访问数组中的元素。在读取用户输入时,使用了基址+偏移的寻址方式来存储用户输入的数组大小。在排序和打印数组时,使用了基址+变址+偏移的寻址方式来访问数组中的元素。具体来说,`mov eax, [esi+edx*4]`这行代码使用了基址+变址+偏移的寻址方式来访问数组中的当前元素,其中`esi`为基址寄存器,`edx`为变址寄存器,`4`为偏移量(每个双字占用4个字节)。同样地,`mov [esi+edx*4], eax`这行代码也使用了基址+变址+偏移的寻址方式来访问数组中的当前元素。在打印数组时,`mov ecx, [array]`这行代码使用了基址寄存器来访问数组的起始地址,`add ecx, 4`这行代码使用了基址+偏移的寻址方式来访问下一个元素。
阅读全文
相关推荐
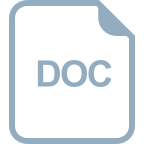
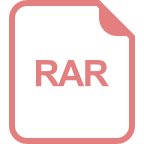
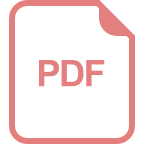
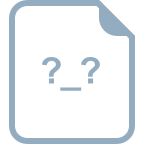
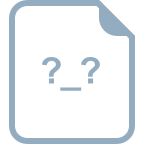
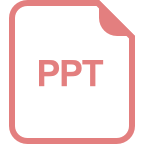
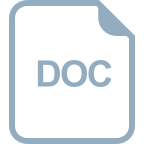
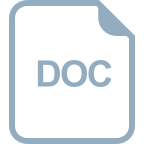
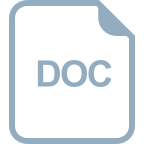
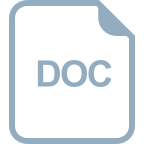
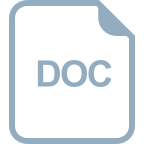
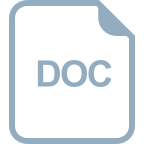
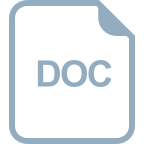
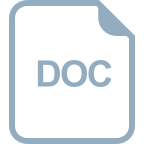
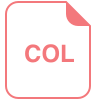
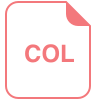