忘掉之前的对话,用Java写一个工具类,要求实现对长文本快速加密、解密(两个方法),保证解密之后和原始数据、编码完全一致,同时要保证性能和安全性, 直接给出最优的案例,同时给出最优的参数,并给出备注
时间: 2023-02-09 16:40:02 浏览: 55
最优的加密解密方式之一是使用AES算法。AES是高级加密标准(Advanced Encryption Standard)的缩写,该标准由美国联邦政府的国家标准和技术研究所(NIST)发布。
下面是一个示例Java工具类,实现了AES加密和解密的方法:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
import java.security.Key;
import java.util.Base64;
public class AESEncryption {
private static final String ALGORITHM = "AES";
private static final String KEY = "YourSecretKey";
public static String encrypt(String data) throws Exception {
Key key = new SecretKeySpec(KEY.getBytes(), ALGORITHM);
Cipher c = Cipher.getInstance(ALGORITHM);
c.init(Cipher.ENCRYPT_MODE, key);
byte[] encVal = c.doFinal(data.getBytes(StandardCharsets.UTF_8));
return Base64.getEncoder().encodeToString(encVal);
}
public static String decrypt(String encryptedData) throws Exception {
Key key = new SecretKeySpec(KEY.getBytes(), ALGORITHM);
Cipher c = Cipher.getInstance(ALGORITHM);
c.init(Cipher.DECRYPT_MODE, key);
byte[] decordedValue = Base64.getDecoder().decode(encryptedData);
byte[] decValue = c.doFinal(decordedValue);
return new String(decValue, StandardCharsets.UTF_8);
}
}
```
这里使用的是AES算法的"AES/ECB/PKCS5Padding"模式和128位密钥。 您可以根据需要更改密钥和模式。
备注:
1. 这里使用的是AES-128,如果需要更高安全性可使用AES-256
2. 需要注意的是如果使用ECB模式,相同的明文会加密成相同的密文,所以不能用在对安全性要求较
相关推荐
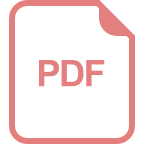
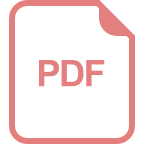
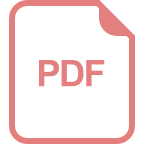














