设计一个控制台地图游戏,玩家需要通过控制台输入来移动自己的角色,并在地图上寻找宝藏。玩家可以通过键盘控制小球向上、向下、向左或向右移动。当小球移动到宝藏处时,游戏结束,输出恭喜信息。 可以通过输入行数和列数调整地图的大小; 可以随机生成宝藏位置和角色起始位置,注 意两者不能相同,借助 rand ()函数生成; 可以采用 w 、 s 、 a 、 d 表示键盘控制的方向。游戏开始时用 O 表示角色起始位置, x 表示宝藏所在位置。当到达目的地后,即 X 和 O 符号重合时变成"$"符号。
时间: 2024-03-17 15:46:02 浏览: 19
好的,这个游戏看起来很有趣!这里是一个简单的实现方式,欢迎参考:
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
const int MAX_ROW = 20;
const int MAX_COL = 20;
const char PLAYER = 'O';
const char TREASURE = 'X';
const char FOUND_TREASURE = '$';
const char WALL = '#';
const char EMPTY = ' ';
void initMap(char map[][MAX_COL], int row, int col);
void printMap(char map[][MAX_COL], int row, int col);
bool movePlayer(char map[][MAX_COL], int row, int col, char input);
bool isTreasureFound(char map[][MAX_COL], int row, int col);
void generateRandomPosition(int& row, int& col, int maxRow, int maxCol);
int main() {
srand(time(NULL)); // 初始化随机数生成器
int row, col;
cout << "请输入地图的行数和列数(不大于" << MAX_ROW << "):";
cin >> row >> col;
if (row > MAX_ROW || col > MAX_COL) {
cout << "输入的行数或列数超过了最大值!" << endl;
return 1;
}
char map[MAX_ROW][MAX_COL];
initMap(map, row, col);
int playerRow, playerCol, treasureRow, treasureCol;
generateRandomPosition(playerRow, playerCol, row, col);
generateRandomPosition(treasureRow, treasureCol, row, col);
while (playerRow == treasureRow && playerCol == treasureCol) {
// 确保宝藏和角色不在同一个位置
generateRandomPosition(treasureRow, treasureCol, row, col);
}
map[playerRow][playerCol] = PLAYER;
map[treasureRow][treasureCol] = TREASURE;
cout << "游戏开始!请用 W、A、S、D 控制角色移动,X 是目标,$ 是你找到目标的位置。" << endl;
printMap(map, row, col);
char input;
while (cin >> input) {
if (movePlayer(map, row, col, input)) {
if (isTreasureFound(map, row, col)) {
map[playerRow][playerCol] = FOUND_TREASURE;
printMap(map, row, col);
cout << "恭喜你找到宝藏了!" << endl;
break;
}
printMap(map, row, col);
} else {
cout << "你不能向那个方向移动!" << endl;
}
}
return 0;
}
// 初始化地图
void initMap(char map[][MAX_COL], int row, int col) {
for (int i = 0; i < row; i++) {
for (int j = 0; j < col; j++) {
if (i == 0 || i == row - 1 || j == 0 || j == col - 1) {
map[i][j] = WALL;
} else {
map[i][j] = EMPTY;
}
}
}
}
// 打印地图
void printMap(char map[][MAX_COL], int row, int col) {
for (int i = 0; i < row; i++) {
for (int j = 0; j < col; j++) {
cout << map[i][j];
}
cout << endl;
}
}
// 移动角色
bool movePlayer(char map[][MAX_COL], int row, int col, char input) {
int playerRow, playerCol;
for (int i = 0; i < row; i++) {
for (int j = 0; j < col; j++) {
if (map[i][j] == PLAYER) {
playerRow = i;
playerCol = j;
}
}
}
switch (input) {
case 'W':
case 'w':
if (map[playerRow - 1][playerCol] != WALL) {
map[playerRow][playerCol] = EMPTY;
map[playerRow - 1][playerCol] = PLAYER;
return true;
} else {
return false;
}
break;
case 'A':
case 'a':
if (map[playerRow][playerCol - 1] != WALL) {
map[playerRow][playerCol] = EMPTY;
map[playerRow][playerCol - 1] = PLAYER;
return true;
} else {
return false;
}
break;
case 'S':
case 's':
if (map[playerRow + 1][playerCol] != WALL) {
map[playerRow][playerCol] = EMPTY;
map[playerRow + 1][playerCol] = PLAYER;
return true;
} else {
return false;
}
break;
case 'D':
case 'd':
if (map[playerRow][playerCol + 1] != WALL) {
map[playerRow][playerCol] = EMPTY;
map[playerRow][playerCol + 1] = PLAYER;
return true;
} else {
return false;
}
break;
default:
return false;
break;
}
}
// 判断是否找到宝藏
bool isTreasureFound(char map[][MAX_COL], int row, int col) {
int playerRow, playerCol, treasureRow, treasureCol;
for (int i = 0; i < row; i++) {
for (int j = 0; j < col; j++) {
if (map[i][j] == PLAYER) {
playerRow = i;
playerCol = j;
}
if (map[i][j] == TREASURE) {
treasureRow = i;
treasureCol = j;
}
}
}
return playerRow == treasureRow && playerCol == treasureCol;
}
// 生成随机位置
void generateRandomPosition(int& row, int& col, int maxRow, int maxCol) {
row = rand() % (maxRow - 2) + 1;
col = rand() % (maxCol - 2) + 1;
}
```
这个游戏可以自定义地图大小,随机生成角色和宝藏的位置,支持键盘控制角色移动,可以判断是否找到宝藏。
相关推荐
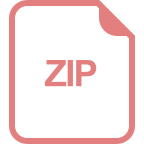
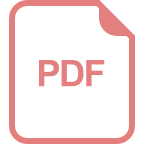







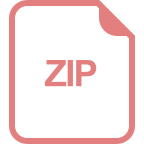
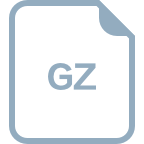
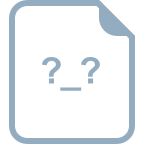