implements Serialization
时间: 2024-01-02 07:10:14 浏览: 82
Serialization is a process of converting the state of an object into a byte stream that can be easily stored or transmitted across a network. To implement serialization in a class, the class needs to implement the Serializable interface provided by Java. This interface is a marker interface with no methods, but it indicates that the class can be serialized.
To implement serialization, the class needs to define a serialVersionUID field to provide version control for the serialized object. The class also needs to override the default serialization methods such as writeObject() and readObject() to customize the serialization process. By default, all non-static and non-transient fields of the class are serialized.
Here is an example of a class implementing serialization:
import java.io.Serializable;
public class Person implements Serializable {
private static final long serialVersionUID = 1L;
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
private void writeObject(java.io.ObjectOutputStream out) throws IOException {
out.writeObject(name);
out.writeInt(age);
}
private void readObject(java.io.ObjectInputStream in) throws IOException, ClassNotFoundException {
name = (String)in.readObject();
age = in.readInt();
}
}
In this example, the Person class implements the Serializable interface and defines a serialVersionUID field. The writeObject() and readObject() methods are also overridden to customize the serialization process. The name and age fields are serialized and deserialized using the respective methods.
阅读全文
相关推荐
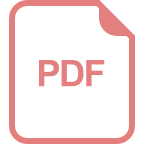
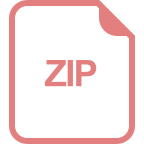
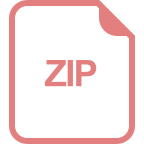
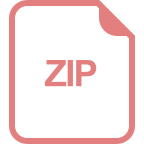
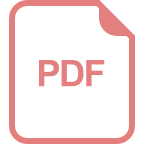
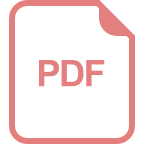






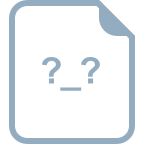