使用scanner输入超时的题目
时间: 2023-08-31 09:33:11 浏览: 84
### 回答1:
题目描述:
给定一个整数n,和n个整数,每个整数都在1到100之间,对于每个整数,输出其质因子分解后因子个数的奇偶性。
输入格式:
第一行包含整数n。
接下来n行,每行包含一个整数。
输出格式:
共n行,每行一个整数,表示其质因子分解后因子个数的奇偶性。
输入样例:
3
2
6
8
输出样例:
1
0
1
代码实现(使用Scanner):
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int n = scanner.nextInt();
while (n-- > 0) {
int x = scanner.nextInt();
int cnt = 0;
for (int i = 2; i <= Math.sqrt(x); i++) {
if (x % i == 0) {
cnt++;
while (x % i == 0) {
x /= i;
}
}
}
if (x > 1) {
cnt++;
}
System.out.println(cnt % 2);
}
}
}
```
上述代码中,我们使用了Scanner类从标准输入中读取数据。当输入数据较多时,可能会出现超时的情况,此时可以考虑使用BufferedReader类进行优化。
### 回答2:
Scanner是Java中的一个类,用于从输入流中读取用户输入的数据。当使用Scanner输入超时时,可以考虑以下几个方法来解决。
方法一:使用线程的join方法
通过创建一个新线程来运行Scanner读取输入的代码,然后设置一个超时时间,当超时时,使用join方法强制结束线程。
```java
import java.util.Scanner;
public class TimeoutExample {
public static void main(String[] args) throws InterruptedException {
Thread thread = new Thread(() -> {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入:");
String input = scanner.nextLine();
System.out.println("你输入的是:" + input);
scanner.close();
});
thread.start();
thread.join(5000); // 设置超时时间为5秒
if (thread.isAlive()) {
thread.interrupt();
System.out.println("输入超时!");
}
}
}
```
方法二:使用ExecutorService的submit方法和Future的get方法
ExecutorService是Java提供的一个用于管理线程池的类,可以使用它的submit方法来提交需要执行的任务,并通过调用Future的get方法设置超时时间。
```java
import java.util.Scanner;
import java.util.concurrent.*;
public class TimeoutExample {
public static void main(String[] args) throws InterruptedException, ExecutionException, TimeoutException {
ExecutorService executor = Executors.newSingleThreadExecutor();
Future<String> future = executor.submit(() -> {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入:");
String input = scanner.nextLine();
System.out.println("你输入的是:" + input);
scanner.close();
return input;
});
try {
String result = future.get(5, TimeUnit.SECONDS); // 设置超时时间为5秒
System.out.println("输入的结果是:" + result);
} catch (TimeoutException e) {
future.cancel(true);
System.out.println("输入超时!");
}
executor.shutdown();
}
}
```
以上两种方法均可以有效解决Scanner输入超时的问题,可以根据具体需求选择适合的方法来使用。
### 回答3:
当使用Scanner输入时,可能会遇到输入超时的情况。这种情况多数是由于用户没有按时输入所导致的。
要解决这个问题,可以通过使用线程的方式来实现输入超时的控制。具体步骤如下:
1. 首先,创建一个Scanner对象用于获取用户输入。
2. 然后,创建一个线程,用于在规定时间内接收用户输入。在该线程中,可以使用Scanner的nextLine()方法来等待用户输入,代码如下:
```
Scanner scanner = new Scanner(System.in);
String input = scanner.nextLine();
```
3. 在主线程中,启动上述创建的线程,并同时开始计时。代码如下:
```
Thread inputThread = new Thread(new Runnable() {
public void run() {
// 向用户显示输入提示
System.out.print("请在5秒内输入:");
// 等待用户输入
input = scanner.nextLine();
}
});
inputThread.start();
// 等待用户输入的线程的时间
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
// 五秒之后,检查用户是否输入
if (input == null) {
System.out.println("时间已过,未接收到输入!");
} else {
System.out.println("你输入的是:" + input);
}
```
4. 最后,根据需求设置适当的输入超时时间。在上述代码中,我们设置了5秒的超时时间。
通过上述步骤,我们可以实现对用户输入超时的控制。当然,在实际使用中,还需要根据具体的需求对代码进行相应的修改和完善。
阅读全文
相关推荐
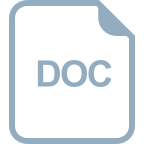
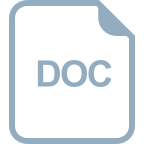
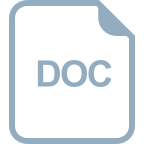



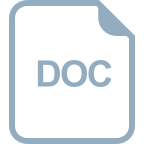
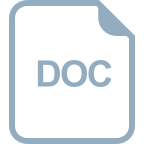
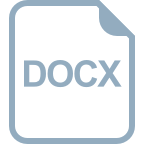
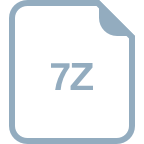
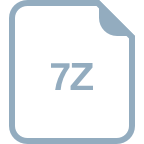
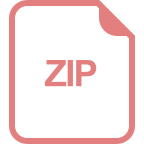
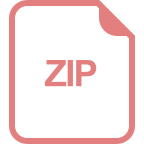
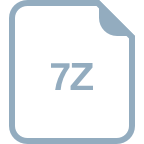