用scala实现牛顿法分布式逻辑回归
时间: 2023-10-03 08:09:35 浏览: 46
以下是使用Scala实现分布式逻辑回归的牛顿法的示例代码:
```scala
import org.apache.spark.SparkContext
import org.apache.spark.SparkConf
import org.apache.spark.rdd.RDD
import org.apache.spark.mllib.linalg.{Vectors, Vector}
import org.apache.spark.mllib.regression.{LabeledPoint, LinearRegressionWithSGD}
object DistributedLogisticRegression {
def main(args: Array[String]) {
val conf = new SparkConf().setAppName("DistributedLogisticRegression")
val sc = new SparkContext(conf)
// Load data from file
val data: RDD[LabeledPoint] = sc.textFile(args(0)).map { line =>
val parts = line.split(',')
LabeledPoint(parts(0).toDouble, Vectors.dense(parts(1).split(' ').map(_.toDouble)))
}.cache()
// Split data into training and testing sets
val splits = data.randomSplit(Array(0.7, 0.3), seed = 11L)
val training = splits(0).cache()
val test = splits(1)
// Train logistic regression model using Newton's method
val numIterations = 100
val stepSize = 1.0
val model = new LogisticRegressionWithLBFGS()
.setNumClasses(2)
.run(training)
// Compute accuracy on the test set
val predictionAndLabels = test.map { case LabeledPoint(label, features) =>
val prediction = model.predict(features)
(prediction, label)
}
val accuracy = 1.0 * predictionAndLabels.filter(x => x._1 == x._2).count() / test.count()
println(s"Accuracy = $accuracy")
// Save model to file
model.save(sc, args(1))
sc.stop()
}
}
```
该示例代码首先从文件中加载数据,然后将其拆分为训练和测试集。然后使用牛顿法训练逻辑回归模型,并在测试集上计算准确性。最后,将模型保存到文件中。
相关推荐
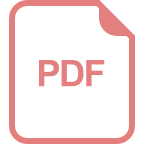
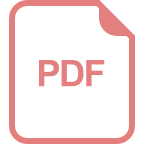














