C 清空文件夹
时间: 2023-08-01 10:09:28 浏览: 292
在 C 语言中,要清空一个文件夹,需要使用系统调用来删除该文件夹中的所有子文件和子文件夹。以下是一个示例代码,可以实现清空指定文件夹的功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <dirent.h>
#include <sys/stat.h>
#include <string.h>
void clearFolder(char *path) {
DIR *dir = opendir(path);
struct dirent *entry;
while ((entry = readdir(dir)) != NULL) {
// 跳过 . 和 ..
if (strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0) {
continue;
}
char *filepath = (char *)malloc(strlen(path) + strlen(entry->d_name) + 2);
sprintf(filepath, "%s/%s", path, entry->d_name);
struct stat statbuf;
if (lstat(filepath, &statbuf) == -1) {
perror("lstat error");
continue;
}
if (S_ISDIR(statbuf.st_mode)) {
// 如果是目录,递归清空目录
clearFolder(filepath);
rmdir(filepath);
} else {
// 如果是文件,直接删除
unlink(filepath);
}
free(filepath);
}
closedir(dir);
}
int main() {
char *path = "/path/to/folder";
clearFolder(path);
return 0;
}
```
在该示例代码中,我们使用 opendir 函数打开指定目录,并使用 readdir 函数遍历目录中的所有子文件和子文件夹。对于每个子文件和子文件夹,我们使用 lstat 函数获取其文件类型和其他属性。如果是目录,我们递归清空目录并使用 rmdir 函数删除该目录;如果是文件,我们直接使用 unlink 函数删除该文件。最后使用 closedir 函数关闭目录。
阅读全文
相关推荐








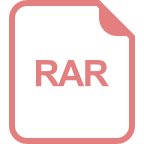





