kotlin中怎么自定义View
时间: 2024-05-04 14:17:51 浏览: 12
在 Kotlin 中自定义 View 的步骤与 Java 中基本相同。下面是一个简单的自定义 View 的示例:
1. 创建一个新的 Kotlin 类,扩展自 View 类。
```
class MyCustomView(context: Context, attrs: AttributeSet?) : View(context, attrs) {
// View 的绘制代码将在这里添加
}
```
2. 实现 `onMeasure()` 方法来定义 View 的大小。在这个方法中,使用 `setMeasuredDimension()` 方法来设置 View 的宽度和高度。
```
override fun onMeasure(widthMeasureSpec: Int, heightMeasureSpec: Int) {
super.onMeasure(widthMeasureSpec, heightMeasureSpec)
val desiredWidth = 100 // 期望的宽度
val desiredHeight = 100 // 期望的高度
val widthMode = MeasureSpec.getMode(widthMeasureSpec)
val widthSize = MeasureSpec.getSize(widthMeasureSpec)
val heightMode = MeasureSpec.getMode(heightMeasureSpec)
val heightSize = MeasureSpec.getSize(heightMeasureSpec)
val width = when (widthMode) {
MeasureSpec.EXACTLY -> widthSize
MeasureSpec.AT_MOST -> min(desiredWidth, widthSize)
else -> desiredWidth
}
val height = when (heightMode) {
MeasureSpec.EXACTLY -> heightSize
MeasureSpec.AT_MOST -> min(desiredHeight, heightSize)
else -> desiredHeight
}
setMeasuredDimension(width, height)
}
```
3. 实现 `onDraw()` 方法来绘制 View。在这个方法中,可以使用 Canvas API 来绘制所需的视图元素。
```
override fun onDraw(canvas: Canvas) {
super.onDraw(canvas)
// 绘制一个圆形
val centerX = width / 2f
val centerY = height / 2f
val radius = min(centerX, centerY)
canvas.drawCircle(centerX, centerY, radius, Paint().apply {
color = Color.BLUE
style = Paint.Style.FILL
})
}
```
4. 在 XML 布局文件中添加自定义 View。
```
<com.example.MyCustomView
android:layout_width="match_parent"
android:layout_height="match_parent" />
```
这样就完成了一个简单的自定义 View。当然,这只是一个简单的示例,实际上自定义 View 的实现可能会更加复杂,具体实现方式取决于您的需求。
相关推荐
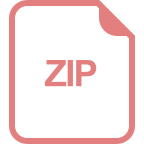
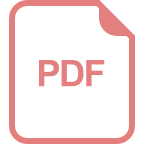
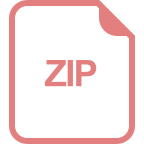














