kotlin自定义view
时间: 2023-07-19 19:45:56 浏览: 75
要在 Kotlin 中创建自定义 View,可以按照以下步骤进行操作:
1. 创建一个继承自 View 的类,并重写构造函数。
```kotlin
class MyCustomView(context: Context, attrs: AttributeSet?) : View(context, attrs) {
// 构造函数
}
```
2. 在构造函数中获取属性值。
```kotlin
class MyCustomView(context: Context, attrs: AttributeSet?) : View(context, attrs) {
private var myAttrValue: Int = 0
init {
// 获取自定义属性值
val typedArray = context.obtainStyledAttributes(attrs, R.styleable.MyCustomView)
myAttrValue = typedArray.getInt(R.styleable.MyCustomView_myAttr, 0)
typedArray.recycle()
}
}
```
3. 实现 onMeasure() 方法来确定 View 的大小。
```kotlin
class MyCustomView(context: Context, attrs: AttributeSet?) : View(context, attrs) {
private var myAttrValue: Int = 0
init {
// 获取自定义属性值
val typedArray = context.obtainStyledAttributes(attrs, R.styleable.MyCustomView)
myAttrValue = typedArray.getInt(R.styleable.MyCustomView_myAttr, 0)
typedArray.recycle()
}
override fun onMeasure(widthMeasureSpec: Int, heightMeasureSpec: Int) {
val width = MeasureSpec.getSize(widthMeasureSpec)
val height = MeasureSpec.getSize(heightMeasureSpec)
setMeasuredDimension(width, height)
}
}
```
4. 实现 onDraw() 方法来绘制 View。
```kotlin
class MyCustomView(context: Context, attrs: AttributeSet?) : View(context, attrs) {
private var myAttrValue: Int = 0
init {
// 获取自定义属性值
val typedArray = context.obtainStyledAttributes(attrs, R.styleable.MyCustomView)
myAttrValue = typedArray.getInt(R.styleable.MyCustomView_myAttr, 0)
typedArray.recycle()
}
override fun onMeasure(widthMeasureSpec: Int, heightMeasureSpec: Int) {
val width = MeasureSpec.getSize(widthMeasureSpec)
val height = MeasureSpec.getSize(heightMeasureSpec)
setMeasuredDimension(width, height)
}
override fun onDraw(canvas: Canvas?) {
super.onDraw(canvas)
// 在 canvas 上绘制 View
}
}
```
5. 可以为自定义 View 定义自己的属性。
```xml
<declare-styleable name="MyCustomView">
<attr name="myAttr" format="integer" />
</declare-styleable>
```
在使用自定义 View 时,可以通过 XML 设置自定义属性的值。
```xml
<com.example.MyCustomView
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:myAttr="10" />
```
相关推荐
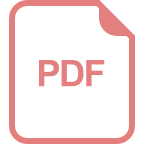
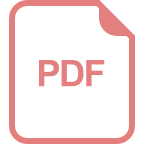
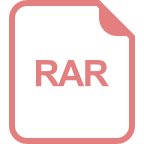














