请给一个正确的多维Quantile regression C++实现代码及案例
时间: 2024-04-23 19:24:43 浏览: 89
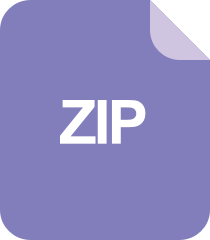
qt的c++源代码案例
以下是一个基于Eigen和boost库实现的多维Quantile regression C++代码示例:
```cpp
#include <iostream>
#include <vector>
#include <Eigen/Dense>
#include <boost/math/distributions/normal.hpp>
using namespace Eigen;
// 多维Quantile regression函数
VectorXd quantile_regression(MatrixXd X, VectorXd y, double tau) {
int n = X.rows(), p = X.cols();
VectorXd beta(p);
beta.setZero();
double epsilon = 1e-6;
double alpha = 0.05;
double z = boost::math::quantile(boost::math::normal_distribution<>(0.0, 1.0), 1 - alpha / 2);
while (true) {
VectorXd y_star = X * beta;
VectorXd e = y - y_star;
VectorXd q(n);
for (int i = 0; i < n; i++) {
if (e(i) >= 0) {
q(i) = tau * e(i);
} else {
q(i) = (tau - 1) * e(i);
}
}
MatrixXd W(n, n);
for (int i = 0; i < n; i++) {
W(i, i) = tau * (1 - tau);
}
MatrixXd XtX = X.transpose() * W * X;
VectorXd Xty = X.transpose() * W * y;
JacobiSVD<MatrixXd> svd(XtX, ComputeThinU | ComputeThinV);
beta = svd.solve(Xty);
double max_diff = (X * beta - y_star).cwiseAbs().maxCoeff();
if (max_diff < epsilon) {
break;
}
}
return beta;
}
int main() {
std::vector<std::vector<double>> x_data = {{1, 2}, {2, 3}, {3, 4}, {4, 5}, {5, 6}, {6, 7}, {7, 8}, {8, 9}, {9, 10}};
std::vector<double> y_data = {2, 3, 4, 5, 6, 7, 8, 9, 10};
int n = y_data.size(), p = x_data[0].size();
MatrixXd X(n, p);
VectorXd y(n);
for (int i = 0; i < n; i++) {
for (int j = 0; j < p; j++) {
X(i, j) = x_data[i][j];
}
y(i) = y_data[i];
}
double tau = 0.5;
VectorXd beta = quantile_regression(X, y, tau);
std::cout << "beta = \n" << beta << std::endl;
return 0;
}
```
这是一个简单的二维Quantile regression的例子,输入数据为X和y,其中X为n行p列的矩阵,每行代表一个样本的p个特征,y为n维向量,代表每个样本的标签。quantile_regression函数输出一个p维向量beta,代表模型的参数。
阅读全文
相关推荐
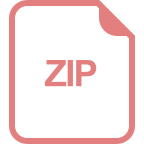

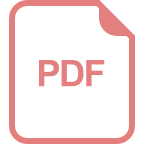
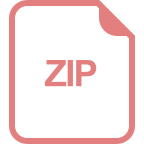
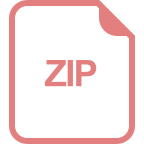
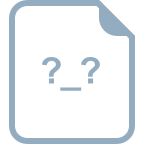
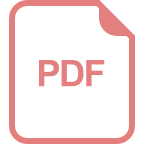
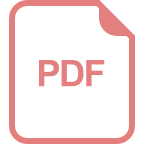
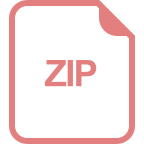
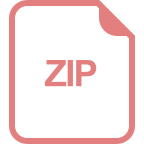
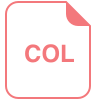

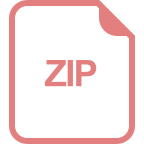

