初始化 unsigned char数组为0
时间: 2024-03-03 10:48:30 浏览: 243
可以使用以下方式初始化 unsigned char 数组为 0:
```c++
unsigned char arr[SIZE] = {0};
```
这样,数组中的每个元素都会被初始化为 0。其中 SIZE 是数组的大小。如果没有指定数组大小,可以使用以下方式:
```c++
unsigned char arr[] = {0};
```
这样编译器会自动根据初始化的元素数量来计算数组大小。
相关问题
用c实现将unsigned char数组里的数据保存为jpg文件
要将unsigned char数组里的数据保存为jpg文件,可以使用libjpeg库。下面是一个简单的C代码示例:
```
#include <stdio.h>
#include <jpeglib.h>
void write_jpeg_file(unsigned char *data, int width, int height, char *filename)
{
struct jpeg_compress_struct cinfo;
struct jpeg_error_mgr jerr;
FILE *outfile = fopen(filename, "wb");
if (!outfile) {
fprintf(stderr, "Can't open %s\n", filename);
return;
}
cinfo.err = jpeg_std_error(&jerr);
jpeg_create_compress(&cinfo);
jpeg_stdio_dest(&cinfo, outfile);
cinfo.image_width = width;
cinfo.image_height = height;
cinfo.input_components = 3;
cinfo.in_color_space = JCS_RGB;
jpeg_set_defaults(&cinfo);
jpeg_start_compress(&cinfo, TRUE);
JSAMPROW row_pointer[1];
while (cinfo.next_scanline < cinfo.image_height) {
row_pointer[0] = & data[cinfo.next_scanline * width * 3];
jpeg_write_scanlines(&cinfo, row_pointer, 1);
}
jpeg_finish_compress(&cinfo);
fclose(outfile);
jpeg_destroy_compress(&cinfo);
}
int main() {
// 假设数据保存在data数组中,宽度为width,高度为height
unsigned char data[width * height * 3];
// 将数据保存为jpg文件
write_jpeg_file(data, width, height, "output.jpg");
return 0;
}
```
在这个示例中,我们定义了一个write_jpeg_file函数,用于将数据保存为jpg文件。函数接受四个参数:数据指针data,宽度width,高度height和文件名filename。在函数中,我们首先打开文件并初始化jpeg压缩器。然后,设置图像的宽度、高度、像素数据格式等参数。接着,我们逐行将像素数据写入文件中,最后关闭文件和压缩器。在main函数中,我们调用write_jpeg_file函数将数据保存为jpg文件。
unsigned char型数组怎么赋值_【C++程序设计】第4期:数组及其应用
要给一个 unsigned char 类型的数组赋值,可以使用循环结构依次给数组中每个元素赋值,也可以使用初始化列表的方式进行赋值。
使用循环结构赋值的代码示例:
```cpp
unsigned char arr[5];
for(int i=0; i<5; i++){
arr[i] = i + 1;
}
```
这段代码会给数组 `arr` 中的元素赋值为 1、2、3、4、5。
使用初始化列表的方式赋值的代码示例:
```cpp
unsigned char arr[5] = {1, 2, 3, 4, 5};
```
这段代码会直接将数组 `arr` 中的元素赋值为 1、2、3、4、5。
阅读全文
相关推荐
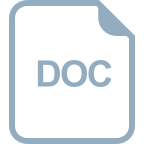
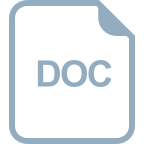
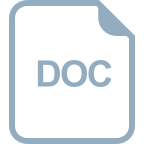













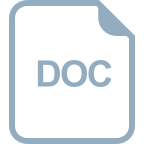