Java 使用 Multimaps.index分组计算一组的总年龄
时间: 2024-05-03 13:20:07 浏览: 134
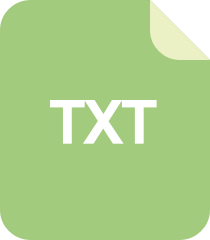
java 判断年龄
您好,我可以帮您解答关于Java中使用Multimaps.index进行分组计算一组的总年龄的问题。
首先需要导入Google Guava库中的Multimaps类。然后,我们可以使用Multimaps.index方法将一组对象按照某个属性进行分组,并将其作为Map返回。例如,如果我们有一个Person对象列表,每个Person对象都有一个age属性,我们可以按照age属性将Person对象列表分组,得到一个Map<Integer, List<Person>>。其中,Map的键为age属性的值,值为具有相同age属性值的Person对象列表。
接下来,我们可以遍历Map中的每一组,计算出该组中所有Person对象的年龄总和即可。具体代码如下所示:
```java
import com.google.common.collect.ArrayListMultimap;
import com.google.common.collect.ListMultimap;
import com.google.common.collect.Multimaps;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
public class Test {
public static void main(String[] args) {
// 创建Person对象列表
List<Person> people = Arrays.asList(
new Person("Alice", 20),
new Person("Bob", 18),
new Person("Charlie", 20),
new Person("David", 22),
new Person("Eva", 18)
);
// 按照年龄属性进行分组
ListMultimap<Integer, Person> ageGroup = Multimaps.index(people, Person::getAge);
// 计算每组的总年龄
for (Map.Entry<Integer, List<Person>> entry : ageGroup.asMap().entrySet()) {
int age = entry.getKey();
List<Person> group = entry.getValue();
int totalAge = group.stream().mapToInt(Person::getAge).sum();
System.out.println("Age " + age + " group: " + group);
System.out.println("Total age: " + totalAge);
}
}
}
class Person {
private final String name;
private final int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
}
```
注意,Multimaps.index方法返回的是一个ListMultimap类型的对象,它可以包含键相同但值不同的映射项,因此适合用于多值映射。如果您只需要单一映射,可以使用Multimaps.uniqueIndex方法。
阅读全文
相关推荐
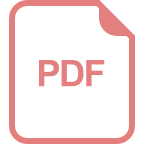

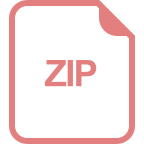
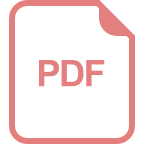
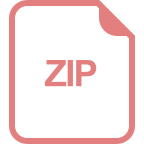
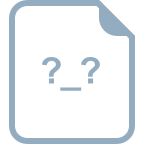
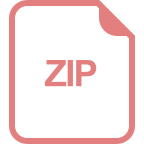
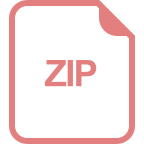
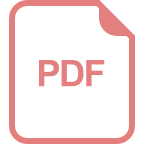
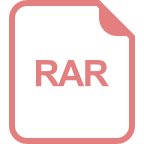
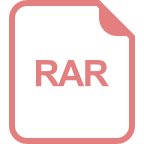
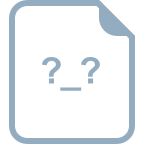
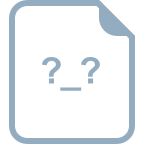
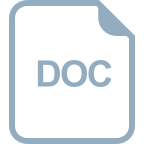
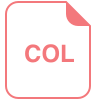
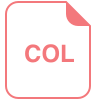
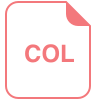