数据结构通讯录C++代码
时间: 2023-07-07 19:17:48 浏览: 78
好的,这是一个使用哈希表实现通讯录的简单C++代码示例:
```c++
#include <iostream>
#include <unordered_map>
#include <string>
using namespace std;
struct ContactInfo {
string phone;
string email;
};
int main() {
unordered_map<string, ContactInfo> contacts;
// 添加联系人
ContactInfo person1 = {"1234567890", "person1@example.com"};
contacts["Person1"] = person1;
ContactInfo person2 = {"0987654321", "person2@example.com"};
contacts["Person2"] = person2;
// 查询联系人
string name = "Person1";
if (contacts.count(name)) {
cout << "Phone: " << contacts[name].phone << endl;
cout << "Email: " << contacts[name].email << endl;
} else {
cout << "Contact not found." << endl;
}
// 删除联系人
contacts.erase("Person2");
return 0;
}
```
在这个示例中,使用了标准库的`unordered_map`来实现哈希表。每个联系人的信息都被封装在`ContactInfo`结构体中,包括电话号码和电子邮件地址。`contacts`哈希表使用人名作为键,联系信息作为值,可以使用`count()`函数来查询是否存在某个联系人,使用`erase()`函数来删除某个联系人。
相关推荐
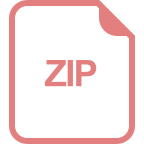
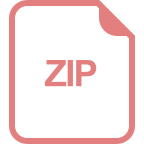















