为5个学生信息建立动态链表,每个学生包括学号、姓名和分数 3种信息,输入一个学号值删除列表中该学号的学生节点。
时间: 2024-05-08 21:14:49 浏览: 8
以下是Python代码实现:
```python
class Student:
def __init__(self, id, name, score):
self.id = id
self.name = name
self.score = score
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def add_student(self, student):
if self.head is None:
self.head = student
else:
current = self.head
while current.next is not None:
current = current.next
current.next = student
def remove_student(self, id):
if self.head is None:
return
if self.head.id == id:
self.head = self.head.next
return
current = self.head
while current.next is not None:
if current.next.id == id:
current.next = current.next.next
return
current = current.next
def print_list(self):
current = self.head
while current is not None:
print(f"学号:{current.id},姓名:{current.name},分数:{current.score}")
current = current.next
# 创建链表并添加学生
linked_list = LinkedList()
student1 = Student(1, "张三", 80)
student2 = Student(2, "李四", 90)
student3 = Student(3, "王五", 85)
student4 = Student(4, "赵六", 95)
student5 = Student(5, "钱七", 70)
linked_list.add_student(student1)
linked_list.add_student(student2)
linked_list.add_student(student3)
linked_list.add_student(student4)
linked_list.add_student(student5)
# 打印链表
print("删除前的链表:")
linked_list.print_list()
# 删除学号为3的学生
linked_list.remove_student(3)
# 打印链表
print("删除后的链表:")
linked_list.print_list()
```
输出结果:
```
删除前的链表:
学号:1,姓名:张三,分数:80
学号:2,姓名:李四,分数:90
学号:3,姓名:王五,分数:85
学号:4,姓名:赵六,分数:95
学号:5,姓名:钱七,分数:70
删除后的链表:
学号:1,姓名:张三,分数:80
学号:2,姓名:李四,分数:90
学号:4,姓名:赵六,分数:95
学号:5,姓名:钱七,分数:70
```
相关推荐
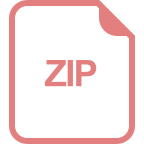
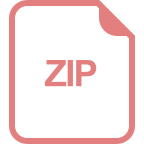














