写一个基于springcloud的电影管理系统
时间: 2023-07-13 17:17:25 浏览: 52
好的。这里简单介绍一下如何搭建一个基于Spring Cloud的电影管理系统。
首先,我们需要创建一个父项目,用于管理各个子模块的依赖。可以在pom.xml中添加如下内容:
```xml
<project>
<groupId>com.example</groupId>
<artifactId>movie-management-system</artifactId>
<version>1.0.0-SNAPSHOT</version>
<packaging>pom</packaging>
<modules>
<module>movie-service</module>
<module>user-service</module>
<module>gateway-service</module>
<module>eureka-service</module>
<module>config-service</module>
</modules>
</project>
```
接下来,我们需要创建各个子模块。其中,`movie-service`模块用于管理电影信息,`user-service`模块用于管理用户信息,`gateway-service`模块用于网关转发请求,`eureka-service`模块用于服务注册和发现,`config-service`模块用于配置管理。
在每个子模块的pom.xml中添加相应依赖:
```xml
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-{service-name}</artifactId>
<version>2.2.6.RELEASE</version>
</dependency>
```
然后,在每个子模块的配置文件中添加相应配置:
1. `movie-service`模块
```yaml
spring:
application:
name: movie-service
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://localhost:3306/movie?useUnicode=true&characterEncoding=utf-8&useSSL=false&serverTimezone=Asia/Shanghai
username: root
password: root
jpa:
hibernate:
ddl-auto: update
```
2. `user-service`模块
```yaml
spring:
application:
name: user-service
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://localhost:3306/user?useUnicode=true&characterEncoding=utf-8&useSSL=false&serverTimezone=Asia/Shanghai
username: root
password: root
jpa:
hibernate:
ddl-auto: update
```
3. `gateway-service`模块
```yaml
spring:
application:
name: gateway-service
cloud:
gateway:
routes:
- id: movie-service
uri: lb://movie-service
predicates:
- Path=/movie/**
- id: user-service
uri: lb://user-service
predicates:
- Path=/user/**
```
4. `eureka-service`模块
```yaml
spring:
application:
name: eureka-service
cloud:
config:
enabled: false
eureka:
instance:
hostname: localhost
client:
register-with-eureka: false
fetch-registry: false
```
5. `config-service`模块
```yaml
spring:
application:
name: config-service
cloud:
config:
server:
git:
uri: https://github.com/{username}/{repository}.git
search-paths: config-repo
```
最后,我们需要在各个模块的启动类上添加注解以启用Spring Cloud的相关功能:
1. `movie-service`模块
```java
@EnableDiscoveryClient
@SpringBootApplication
public class MovieServiceApplication {
public static void main(String[] args) {
SpringApplication.run(MovieServiceApplication.class, args);
}
}
```
2. `user-service`模块
```java
@EnableDiscoveryClient
@SpringBootApplication
public class UserServiceApplication {
public static void main(String[] args) {
SpringApplication.run(UserServiceApplication.class, args);
}
}
```
3. `gateway-service`模块
```java
@EnableDiscoveryClient
@SpringBootApplication
public class GatewayServiceApplication {
public static void main(String[] args) {
SpringApplication.run(GatewayServiceApplication.class, args);
}
}
```
4. `eureka-service`模块
```java
@EnableEurekaServer
@SpringBootApplication
public class EurekaServiceApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaServiceApplication.class, args);
}
}
```
5. `config-service`模块
```java
@EnableConfigServer
@SpringBootApplication
public class ConfigServiceApplication {
public static void main(String[] args) {
SpringApplication.run(ConfigServiceApplication.class, args);
}
}
```
以上就是一个基于Spring Cloud的电影管理系统的搭建过程。需要注意的是,这里只是一个简单的示例,实际的系统中可能还需要考虑更多的因素,如安全性、性能等。
相关推荐
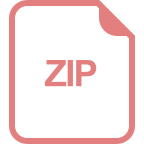
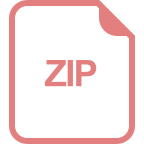
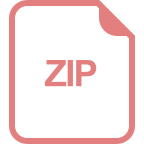














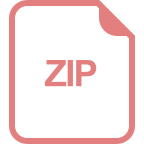