构建一个基于注意力机制的CNN-LSTM回归模型,描述网络的具体结构并给出代码
时间: 2023-06-02 19:01:47 浏览: 84
以下是一个基于注意力机制的CNN-LSTM回归模型的代码示例:
```python
from keras.models import Model
from keras.layers import Input, Dense, LSTM, TimeDistributed, Dropout, Flatten, Conv2D, MaxPooling2D, Permute, Reshape, multiply
# 定义输入形状
input_shape = (32, 32, 3) # 32x32的RGB图像
# 定义CNN部分
input_tensor = Input(shape=input_shape)
x = Conv2D(32, (3, 3), padding='same', activation='relu')(input_tensor)
x = MaxPooling2D(pool_size=(2, 2))(x)
x = Conv2D(64, (3, 3), padding='same', activation='relu')(x)
x = MaxPooling2D(pool_size=(2, 2))(x)
x = Conv2D(128, (3, 3), padding='same', activation='relu')(x)
x = MaxPooling2D(pool_size=(2, 2))(x)
x = Flatten()(x)
cnn_output = Dropout(0.5)(x)
# 定义LSTM部分
lstm_input = Input(shape=(None, 256)) # CNN部分输出的特征长度为256
x = LSTM(128, return_sequences=True)(lstm_input)
x = LSTM(64, return_sequences=True)(x)
x = LSTM(32)(x)
lstm_output = Dropout(0.5)(x)
# 定义注意力机制
attention = Dense(1, activation='tanh')(lstm_output)
attention = Permute((2, 1))(attention)
attention = Reshape((-1,))(attention)
attention = Dense(32, activation='softmax')(attention)
attention = Reshape((1, -1))(attention)
attention = multiply([lstm_output, attention])
attention = LSTM(32)(attention)
# 定义输出层
output = Dense(1, activation='linear')(attention)
# 定义模型
model = Model(inputs=[input_tensor, lstm_input], outputs=output)
model.compile(loss='mean_squared_error', optimizer='adam')
```
以上代码中,首先定义了输入形状为32x32的RGB图像,然后通过卷积神经网络(CNN)提取出图像特征,输出长度为256。接着,定义了一个LSTM层,将CNN输出的特征作为输入,经过多层LSTM后输出一个32维的向量。然后,定义了一个注意力机制,将LSTM输出的向量作为输入,在LSTM的最后一层中进行加权平均,得到一个32维的向量。最后,通过一个全连接层将32维向量映射到一个实数输出。模型的损失函数为均方误差,优化器为Adam。
该模型的输入是一个图像序列和一个LSTM输入序列,输出是一个实数,用于回归任务。
相关推荐
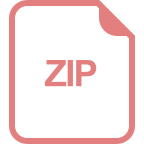
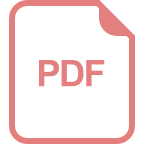















