已知树结构已经从接口中取出来,帮我用react+typescript+AntDesign的开发方式实现可以搜索过滤已知树形结构的功能
时间: 2024-03-13 11:48:17 浏览: 18
好的,我会尽力帮您解决问题。
首先,我们需要先在React中创建一个组件来展示树形结构,可以使用Ant Design的Tree组件。
然后,我们需要在组件中添加一个搜索框,让用户可以输入关键词来过滤树形结构。
最后,我们需要编写过滤函数,对树形结构进行过滤并展示符合要求的节点。
以下是一个简单的实现示例:
```typescript
import React, { useState } from "react";
import { Tree, Input } from "antd";
import { SearchOutlined } from "@ant-design/icons";
import { TreeProps } from "antd/lib/tree";
const { Search } = Input;
interface ITreeNode {
title: string;
key: string;
children?: ITreeNode[];
}
interface IProps extends TreeProps {
treeData: ITreeNode[];
}
const FilterTree: React.FC<IProps> = ({ treeData, ...props }) => {
const [expandedKeys, setExpandedKeys] = useState<string[]>([]);
const [searchValue, setSearchValue] = useState("");
const onExpand = (expandedKeys: string[]) => {
setExpandedKeys(expandedKeys);
};
const onChange = (e: React.ChangeEvent<HTMLInputElement>) => {
const { value } = e.target;
setSearchValue(value);
};
const filterTree = (data: ITreeNode[], keyword: string): ITreeNode[] => {
return data.reduce((acc: ITreeNode[], node: ITreeNode) => {
if (node.title.toLowerCase().includes(keyword.toLowerCase())) {
acc.push(node);
} else if (node.children) {
const children = filterTree(node.children, keyword);
if (children.length) {
acc.push({ ...node, children });
}
}
return acc;
}, []);
};
const filteredData = filterTree(treeData, searchValue);
return (
<>
<Search
placeholder="Search"
prefix={<SearchOutlined />}
onChange={onChange}
style={{ marginBottom: 8 }}
/>
<Tree
{...props}
expandedKeys={expandedKeys}
onExpand={onExpand}
treeData={filteredData}
/>
</>
);
};
export default FilterTree;
```
上述代码中,我们定义了一个 `FilterTree` 组件,其中包含一个搜索框和一个Ant Design的Tree组件。我们使用 `useState` 来管理展开的节点和搜索关键字,然后编写了一个 `filterTree` 函数来过滤树形结构。
在 `onChange` 函数中,我们使用 `setSearchValue` 更新搜索关键字,并在 `filterTree` 函数中根据搜索关键字来过滤节点。
最后,我们将过滤后的节点作为 `Tree` 组件的数据源,渲染出符合要求的节点。
使用时,我们只需要将树形结构传入 `FilterTree` 组件中即可:
```typescript
const treeData = [
{
title: "Node 1",
key: "1",
children: [
{
title: "Child Node 1",
key: "1-1",
},
{
title: "Child Node 2",
key: "1-2",
},
],
},
{
title: "Node 2",
key: "2",
children: [
{
title: "Child Node 3",
key: "2-1",
},
{
title: "Child Node 4",
key: "2-2",
},
],
},
];
<FilterTree treeData={treeData} />
```
这样就可以实现搜索过滤已知树形结构的功能了。
相关推荐
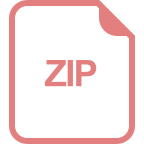
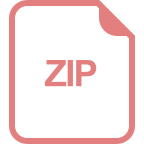
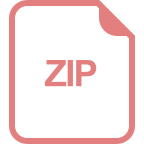














