定义正方体类Cube、长方体类Cuboid、球体类Sphere和圆锥体类Cone及必要的成员。例如正方体需要存储边长信息、长方体需要存储长宽高信息、球体需要存储半径信息、圆锥体需要存储底面半径和高信息。 再定义一个求面积的工具类Volume,该类有多个版本的重载函数calVolume可以分别用于求正方体、长方体、球体和圆锥体对象的体积。main函数已经完成,请根据main函数的内容完成以上所有类的定义。 在程序中,请使用下面的圆周率定义并使用该圆周率进行计算: const double PI=3.14159265; main函数如下: int main(){ double length,width,height,radius; cin>>length; Cube cube(length); cin>>length>>width>>height; Cuboid cuboid(length,width,height); cin>>radius; Sphere sphere(radius); cin>>radius>>height; Cone cone(radius,height); cout<<Volume::calVolume(cube)<<endl; cout<<Volume::calVolume(cuboid)<<endl; cout<<Volume::calVolume(sphere)<<endl; cout<<Volume::calVolume(cone)<<endl; return 0; }
时间: 2024-03-10 18:51:14 浏览: 219
以下是Cube、Cuboid、Sphere和Cone类的定义:
```
#include <iostream>
#include <cmath>
using namespace std;
const double PI = 3.14159265;
// 正方体类
class Cube {
private:
double length; // 边长
public:
Cube(double l) {
length = l;
}
double getLength() {
return length;
}
};
// 长方体类
class Cuboid {
private:
double length; // 长
double width; // 宽
double height; // 高
public:
Cuboid(double l, double w, double h) {
length = l;
width = w;
height = h;
}
double getLength() {
return length;
}
double getWidth() {
return width;
}
double getHeight() {
return height;
}
};
// 球体类
class Sphere {
private:
double radius; // 半径
public:
Sphere(double r) {
radius = r;
}
double getRadius() {
return radius;
}
};
// 圆锥体类
class Cone {
private:
double radius; // 底面半径
double height; // 高
public:
Cone(double r, double h) {
radius = r;
height = h;
}
double getRadius() {
return radius;
}
double getHeight() {
return height;
}
};
```
接下来定义Volume类,用于计算各种几何体的体积:
```
class Volume {
public:
// 计算正方体体积
static double calVolume(Cube cube) {
double length = cube.getLength();
return length * length * length;
}
// 计算长方体体积
static double calVolume(Cuboid cuboid) {
double length = cuboid.getLength();
double width = cuboid.getWidth();
double height = cuboid.getHeight();
return length * width * height;
}
// 计算球体体积
static double calVolume(Sphere sphere) {
double radius = sphere.getRadius();
return 4.0 / 3.0 * PI * pow(radius, 3);
}
// 计算圆锥体体积
static double calVolume(Cone cone) {
double radius = cone.getRadius();
double height = cone.getHeight();
return 1.0 / 3.0 * PI * pow(radius, 2) * height;
}
};
```
最后,在main函数中创建各种几何体对象,并且调用Volume类的静态函数成员calVolume计算体积,输出结果即可。
阅读全文
相关推荐
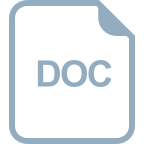
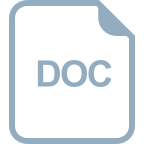
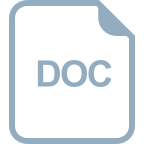


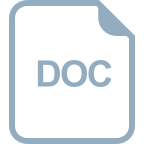
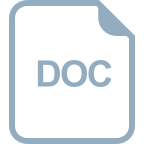
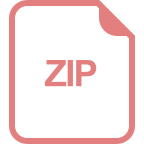