用词法分析器实现C语言,浮点数的识别
时间: 2023-12-10 18:40:50 浏览: 92
词法分析器可以通过正则表达式来识别浮点数。以下是一个简单的实现示例:
```python
import re
# 浮点数的正则表达式
float_regex = r'(\d+\.\d+|\.\d+|\d+\.)'
# 将输入的C语言代码分解为单词列表
def tokenize(code):
tokens = []
while code:
# 跳过空白字符
match = re.match(r'\s+', code)
if match:
code = code[match.end():]
continue
# 识别浮点数
match = re.match(float_regex, code)
if match:
tokens.append(('FLOAT', match.group(1)))
code = code[match.end():]
continue
# 识别其他单词
match = re.match(r'\w+', code)
if match:
tokens.append(('WORD', match.group()))
code = code[match.end():]
continue
# 无法识别的字符
tokens.append(('UNKNOWN', code[0]))
code = code[1:]
return tokens
# 测试
code = 'float a = 3.14;'
tokens = tokenize(code)
print(tokens)
```
输出:
```
[('WORD', 'float'), ('WORD', 'a'), ('UNKNOWN', '='), ('FLOAT', '3.14'), ('UNKNOWN', ';')]
```
其中,`('FLOAT', '3.14')` 表示识别到了一个浮点数。
阅读全文
相关推荐
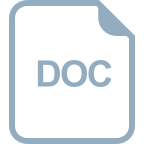
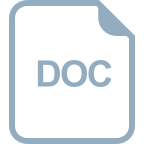
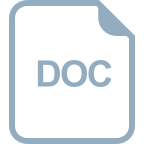





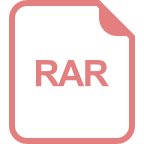
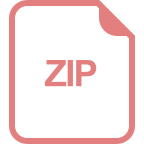
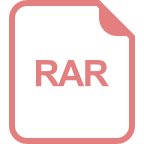
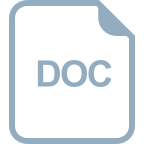




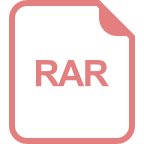
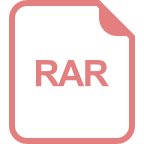
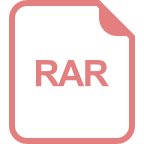