词法分析器代码c语言
时间: 2023-10-29 13:03:05 浏览: 130
词法分析器是编译器的重要组成部分,用于将源代码转换为词法单元。以下是一个简单的词法分析器的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define MAX_LENGTH 100
typedef enum {
INT,
FLOAT,
IDENTIFIER,
ADD_OP,
SUB_OP,
MUL_OP,
DIV_OP,
ASSIGN_OP,
SEMICOLON,
INVALID
} TokenType;
typedef struct {
TokenType type;
char lexeme[MAX_LENGTH];
} Token;
Token getNextToken(FILE* fp) {
Token token;
char ch;
int lexemeIndex = 0;
token.type = INVALID;
// 忽略空格和换行符
while ((ch = fgetc(fp)) != EOF && isspace(ch));
if (ch == EOF) {
token.lexeme[0] = '\0';
return token;
}
// 处理整数和浮点数
if (isdigit(ch)) {
token.type = INT;
while (isdigit(ch)) {
if (lexemeIndex >= MAX_LENGTH - 1) {
printf("Error: Token length exceeds the maximum limit.\n");
exit(1);
}
token.lexeme[lexemeIndex++] = ch;
ch = fgetc(fp);
}
if (ch == '.') {
token.type = FLOAT;
token.lexeme[lexemeIndex++] = ch;
ch = fgetc(fp);
while (isdigit(ch)) {
if (lexemeIndex >= MAX_LENGTH - 1) {
printf("Error: Token length exceeds the maximum limit.\n");
exit(1);
}
token.lexeme[lexemeIndex++] = ch;
ch = fgetc(fp);
}
}
token.lexeme[lexemeIndex] = '\0';
ungetc(ch, fp);
return token;
}
// 处理标识符和关键字
if (isalpha(ch)) {
while (isalnum(ch)) {
if (lexemeIndex >= MAX_LENGTH - 1) {
printf("Error: Token length exceeds the maximum limit.\n");
exit(1);
}
token.lexeme[lexemeIndex++] = ch;
ch = fgetc(fp);
}
token.lexeme[lexemeIndex] = '\0';
ungetc(ch, fp);
// 检查是否是关键字
if (strcmp(token.lexeme, "if") == 0 || strcmp(token.lexeme, "else") == 0 || strcmp(token.lexeme, "while") == 0) {
token.type = KEYWORD;
} else {
token.type = IDENTIFIER;
}
return token;
}
// 处理操作符和分号
switch (ch) {
case '+':
token.type = ADD_OP;
break;
case '-':
token.type = SUB_OP;
break;
case '*':
token.type = MUL_OP;
break;
case '/':
token.type = DIV_OP;
break;
case '=':
token.type = ASSIGN_OP;
break;
case ';':
token.type = SEMICOLON;
break;
}
token.lexeme[0] = ch;
token.lexeme[1] = '\0';
return token;
}
int main() {
FILE* fp = fopen("input.txt", "r");
Token token;
if (fp == NULL) {
printf("Error: Failed to open the input file.\n");
return 1;
}
while ((token = getNextToken(fp)).type != INVALID) {
printf("Type: %d, Lexeme: %s\n", token.type, token.lexeme);
}
fclose(fp);
return 0;
}
```
以上的代码实现了一个简单的词法分析器,能够识别整数、浮点数、标识符、运算符和分号。
阅读全文
相关推荐
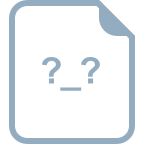
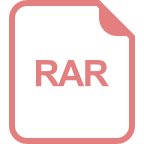
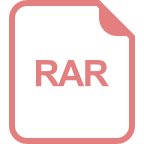
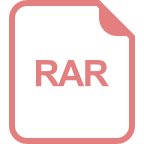
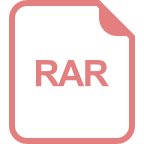
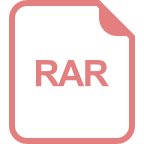
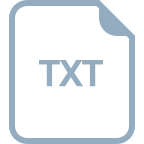
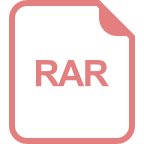
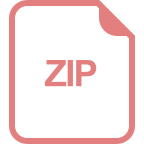
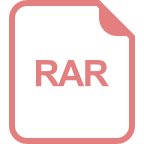
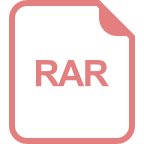
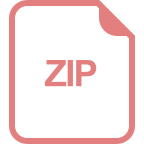