写一段词法分析器的C语言代码
时间: 2023-10-28 18:06:11 浏览: 38
以下是一个简单的词法分析器的C语言代码示例:
```
#include <stdio.h>
#include <ctype.h>
#include <string.h>
// 定义关键字
char *keywords[] = { "auto", "break", "case", "char", "const", "continue", "default",
"do", "double", "else", "enum", "extern", "float", "for", "goto",
"if", "int", "long", "register", "return", "short", "signed",
"sizeof", "static", "struct", "switch", "typedef", "union",
"unsigned", "void", "volatile", "while" };
// 判断是否为关键字
int is_keyword(char *str) {
int i;
int num_keywords = sizeof(keywords) / sizeof(keywords[0]);
for (i = 0; i < num_keywords; i++) {
if (strcmp(str, keywords[i]) == 0) {
return 1;
}
}
return 0;
}
// 词法分析函数
void lex(char *input) {
int i = 0;
int len = strlen(input);
char buffer[100];
while (i < len) {
// 处理空格
while (isspace(input[i])) {
i++;
}
// 处理标识符和关键字
if (isalpha(input[i])) {
int j = 0;
while (isalnum(input[i])) {
buffer[j++] = input[i++];
}
buffer[j] = '\0';
if (is_keyword(buffer)) {
printf("%s is a keyword\n", buffer);
} else {
printf("%s is an identifier\n", buffer);
}
}
// 处理数字
if (isdigit(input[i])) {
int j = 0;
while (isdigit(input[i])) {
buffer[j++] = input[i++];
}
buffer[j] = '\0';
printf("%s is a number\n", buffer);
}
// 处理运算符
if (input[i] == '+' || input[i] == '-' || input[i] == '*' || input[i] == '/' || input[i] == '=') {
printf("%c is an operator\n", input[i]);
i++;
}
// 处理其他字符
if (!isspace(input[i]) && !isalpha(input[i]) && !isdigit(input[i]) && input[i] != '+' && input[i] != '-' && input[i] != '*' && input[i] != '/' && input[i] != '=') {
printf("%c is not a valid character\n", input[i]);
i++;
}
}
}
int main() {
char input[100];
printf("Enter input string: ");
fgets(input, 100, stdin);
lex(input);
return 0;
}
```
该程序可以从标准输入读取一个字符串,并对其进行词法分析,输出识别出的标识符、关键字、数字、运算符和无效字符。
相关推荐
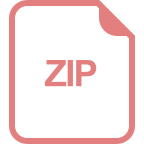
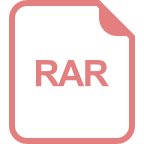














