编译原理词法分析c语言
时间: 2023-10-23 16:13:17 浏览: 122
C语言的词法分析是编译器的第一个阶段,主要任务是将源代码中的字符序列转换成有意义的单词序列(Token)。下面是C语言词法分析的主要过程:
1. 字符扫描
编译器先扫描源代码文件中的字符序列,去除其中的空格、制表符、回车等无关字符,只保留与程序运行有关的字符。
2. 字符分类
编译器将扫描得到的字符序列分类,分为标识符、关键字、运算符、界符等几类。
3. 单词构造
编译器将连续的字符序列构造成一个完整的单词(Token),并为每个单词打上相应的标记,标记通常是一个整数或枚举类型的值。
4. 错误处理
如果编译器在词法分析的过程中发现了不符合规范的字符序列,就会产生一个词法错误(Lexical Error),并给出相应的错误提示信息。
5. 输出单词序列
最后,编译器将得到的单词序列输出给语法分析器,作为其输入。
以上就是C语言词法分析的主要过程,其中比较重要的是字符分类和单词构造。字符分类是将字符序列分为不同的类别,单词构造是将连续的字符序列构造成一个完整的单词。
相关问题
编译原理词法分析C语言实现直接输入进行分析
词法分析是编译原理中的一个基本步骤,它的任务是读入源程序的字符序列,将它们组织成有意义的词素序列,即分词。在C语言实现中,词法分析器通常会使用有限自动机(Finite Automaton)的概念,将输入的字符流转换为词法单元(Token)的集合。
一个简单的C语言实现的词法分析器可能包括以下几个步骤:
1. 读入源代码:程序首先需要从标准输入或其他输入源读取C语言的源代码。
2. 预处理:处理预处理指令(如宏定义、文件包含等)。
3. 字符分类:将源代码中的字符分类,如标识符、数字、关键字、运算符和空白字符等。
4. 状态机设计:设计一个状态机,这个状态机根据输入的字符序列和当前状态,转移到下一个状态,并最终识别出一个个词法单元。
5. 词法单元生成:状态机每识别出一个词法单元,就生成对应的Token,并输出这个Token。
6. 错误处理:遇到无法识别的字符序列时,词法分析器应当报告错误。
例如,一个简单的C语言词法分析器伪代码可能如下所示:
```c
// 假设输入已经通过预处理,并且是字符数组形式
char* source_code = "..."; // 源代码字符串
char current_char = source_code[0]; // 当前处理的字符
int state = 0; // 状态机的初始状态
while(current_char != '\0') { // 遍历源代码直到结束
switch(state) {
case INITIAL: // 初始状态
if(is_keyword(current_char)) {
state = IDENTIFIER; // 转移到标识符状态
} else if(is_digit(current_char)) {
state = NUMBER; // 转移到数字状态
}
// ... 其他状态转移逻辑
break;
// ... 其他状态的处理逻辑
default:
// 如果遇到错误,报告错误并处理
report_error("无效的字符");
break;
}
// 更新当前字符,进入下一个状态
current_char = source_code[next_position++];
}
```
在上述代码中,`is_keyword` 和 `is_digit` 是假设的辅助函数,用于判断当前字符是否是关键字或者数字的一部分。`report_error` 是用于报告错误的函数。实际实现会更加复杂,需要考虑所有的词法单元类型和相应的状态转移逻辑。
编译原理词法分析器c语言
编译原理中的词法分析器是编译器的第一个阶段,也称为扫描器。它的主要任务是将源代码中的字符序列转换为有意义的单词序列,即词法单元(Token)序列。在C语言中,词法单元包括关键字、标识符、常量、运算符、界符等。
C语言的词法分析器通常使用有限状态自动机(Finite State Automaton,FSA)来实现。FSA是一种抽象的计算模型,它可以接受一个输入序列,并根据预先定义好的状态转移规则进行状态转移,最终输出一个结果。
在C语言中,词法分析器需要处理的主要内容包括:
1. 关键字:C语言中有32个关键字,如if、else、while等。
2. 标识符:标识符是程序员定义的变量名、函数名等,由字母、数字和下划线组成,且不能以数字开头。
3. 常量:常量包括整型常量、浮点型常量、字符常量和字符串常量等。
4. 运算符:C语言中有多种运算符,如算术运算符、关系运算符、逻辑运算符等。
5. 界符:C语言中的界符包括括号、分号、花括号等。
C语言的词法分析器通常使用正则表达式来描述各种词法单元的模式,并将其转换为有限状态自动机。在实现过程中,可以使用Lex工具来生成词法分析器的代码。
阅读全文
相关推荐
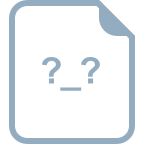
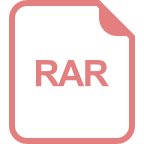
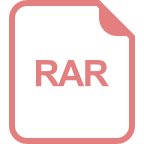
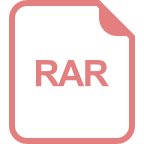




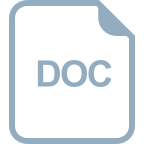

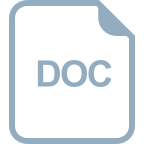

