Write the code about Readers-Writer Problem Implementation in C++.1、 A data set is shared among several concurrent processes.Problem – allow multiple readers (at most 8) to read at the same time. Only one single writer can access the shared data at the same time.
时间: 2024-02-01 15:02:45 浏览: 71
Here is an implementation of Readers-Writer Problem in C++ using Semaphores:
```cpp
#include <iostream>
#include <thread>
#include <mutex>
#include <semaphore.h>
using namespace std;
const int MAX_READERS = 8;
int data = 0;
int active_readers = 0;
bool writer_active = false;
sem_t mutex_read, mutex_write, reader_turnstile, writer_turnstile;
void reader() {
sem_wait(&reader_turnstile);
sem_wait(&mutex_read);
active_readers++;
if (active_readers == 1) {
sem_wait(&writer_turnstile);
}
sem_post(&mutex_read);
sem_post(&reader_turnstile);
cout << "Reader " << this_thread::get_id() << " read data: " << data << endl;
sem_wait(&mutex_read);
active_readers--;
if (active_readers == 0) {
sem_post(&writer_turnstile);
}
sem_post(&mutex_read);
}
void writer() {
sem_wait(&writer_turnstile);
writer_active = true;
sem_post(&writer_turnstile);
sem_wait(&mutex_write);
cout << "Writer " << this_thread::get_id() << " is writing data" << endl;
data++;
cout << "Writer " << this_thread::get_id() << " finished writing data" << endl;
sem_post(&mutex_write);
sem_wait(&writer_turnstile);
writer_active = false;
sem_post(&writer_turnstile);
}
int main() {
sem_init(&mutex_read, 0, 1);
sem_init(&mutex_write, 0, 1);
sem_init(&reader_turnstile, 0, MAX_READERS);
sem_init(&writer_turnstile, 0, 1);
thread readers[MAX_READERS];
thread writers[3];
for (int i = 0; i < MAX_READERS; i++) {
readers[i] = thread(reader);
}
for (int i = 0; i < 3; i++) {
writers[i] = thread(writer);
}
for (int i = 0; i < MAX_READERS; i++) {
readers[i].join();
}
for (int i = 0; i < 3; i++) {
writers[i].join();
}
sem_destroy(&mutex_read);
sem_destroy(&mutex_write);
sem_destroy(&reader_turnstile);
sem_destroy(&writer_turnstile);
return 0;
}
```
In this implementation, we have two semaphores for mutex (one for readers and one for writers) and two turnstile semaphores (one for readers and one for writers).
When a reader wants to read, it first waits on the reader turnstile to ensure that there are not too many readers. Then, it waits on the reader mutex to ensure exclusive access to the shared data's active readers counter. It increments the active readers counter and, if it is the first reader, waits on the writer turnstile to block any writers from accessing the shared data. It then releases the reader mutex and signals the reader turnstile. It reads the shared data and then waits on the reader mutex again to decrement the active readers counter. If it is the last reader, it signals the writer turnstile to allow any waiting writers to access the shared data.
When a writer wants to write, it waits on the writer turnstile to ensure exclusive access to the shared data. It sets the writer_active flag to true, waits on the writer mutex to ensure exclusive access to the shared data, writes to the data, and then sets the writer_active flag to false. It then signals the writer turnstile to allow other writers or readers to access the shared data.
阅读全文
相关推荐
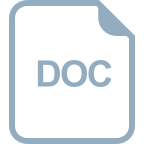
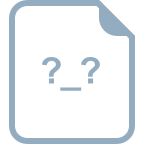


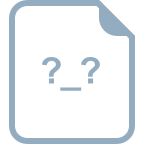
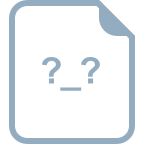
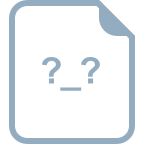
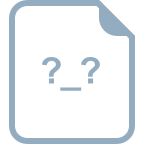
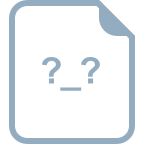
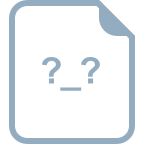
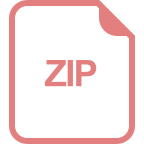
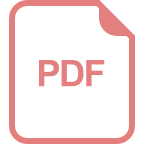