Springboot 定时抽取一张表的数据插入到另一张表实现
时间: 2023-09-24 16:10:51 浏览: 95
可以通过在Spring Boot中使用定时任务来实现定时抽取一张表的数据插入到另一张表。以下是一个基本的示例:
1. 首先,需要在Spring Boot中配置定时任务。可以通过在启动类上添加@EnableScheduling注解来启用定时任务。
```java
@SpringBootApplication
@EnableScheduling
public class MyApp {
public static void main(String[] args) {
SpringApplication.run(MyApp.class, args);
}
}
```
2. 然后,需要创建一个定时任务类,并在其中编写抽取数据并插入到另一张表的逻辑。可以使用Spring Boot提供的@Scheduled注解来指定任务的执行时间。
```java
@Component
public class DataTransferTask {
@Autowired
private SourceRepository sourceRepository;
@Autowired
private TargetRepository targetRepository;
@Scheduled(cron = "0 0 0 * * ?") // 每天凌晨执行
public void transferData() {
List<SourceData> sourceDataList = sourceRepository.findAll();
List<TargetData> targetDataList = new ArrayList<>();
for (SourceData sourceData : sourceDataList) {
TargetData targetData = new TargetData();
// TODO:根据需求对数据进行转换
targetDataList.add(targetData);
}
targetRepository.saveAll(targetDataList);
}
}
```
3. 最后,需要创建源表和目标表的实体类,并分别创建对应的Repository。
```java
@Entity
public class SourceData {
// TODO:定义源表的字段
}
@Entity
public class TargetData {
// TODO:定义目标表的字段
}
@Repository
public interface SourceRepository extends JpaRepository<SourceData, Long> {
}
@Repository
public interface TargetRepository extends JpaRepository<TargetData, Long> {
}
```
通过以上步骤,就可以实现定时抽取一张表的数据插入到另一张表的功能了。需要注意的是,在实际开发中,可能需要根据具体需求对代码进行修改和优化。
相关推荐
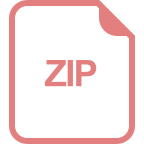
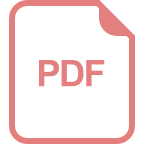














