js 写一个函数,参数是new Date()类型,返回格式为:2020-01-01 00:00:00
时间: 2023-09-03 22:25:16 浏览: 101
### 回答1:
```
function formatDate(date) {
var year = date.getFullYear();
var month = (1 + date.getMonth()).toString().padStart(2, '0');
var day = date.getDate().toString().padStart(2, '0');
var hour = date.getHours().toString().padStart(2, '0');
var minute = date.getMinutes().toString().padStart(2, '0');
var second = date.getSeconds().toString().padStart(2, '0');
return year + '-' + month + '-' + day + ' ' + hour + ':' + minute + ':' + second;
}
```
这是一个 JavaScript 函数,参数是 `Date` 类型,返回格式为:`2020-01-01 00:00:00`。
其中 getFullYear() 获取年份,getMonth() 获取月份,getDate() 获取日期,getHours() 获取小时,getMinutes() 获取分钟,getSeconds() 获取秒数,用padStart(2,'0') 把数字格式化为两位数。
### 回答2:
可以使用 JavaScript 的 Date 对象提供的方法,来完成这个函数的编写。具体步骤如下:
1. 定义一个函数,例如 `formatDate`,它接受一个 `Date` 类型的参数。
2. 在函数内部,使用 `Date` 对象的方法获取传入日期的年、月、日、时、分、秒,分别存储在对应的变量中。
```javascript
const year = date.getFullYear();
const month = String(date.getMonth() + 1).padStart(2, '0');
const day = String(date.getDate()).padStart(2, '0');
const hours = String(date.getHours()).padStart(2, '0');
const minutes = String(date.getMinutes()).padStart(2, '0');
const seconds = String(date.getSeconds()).padStart(2, '0');
```
注意月份要加 1,因为 `getMonth()` 返回的是从 0 开始的索引。
使用 `padStart()` 方法添加前导零,保证每个数值都是两位数。
3. 最后,将这些变量拼接起来,返回格式化后的日期。
```javascript
return `${year}-${month}-${day} ${hours}:${minutes}:${seconds}`;
```
完整的代码如下:
```javascript
function formatDate(date) {
const year = date.getFullYear();
const month = String(date.getMonth() + 1).padStart(2, '0');
const day = String(date.getDate()).padStart(2, '0');
const hours = String(date.getHours()).padStart(2, '0');
const minutes = String(date.getMinutes()).padStart(2, '0');
const seconds = String(date.getSeconds()).padStart(2, '0');
return `${year}-${month}-${day} ${hours}:${minutes}:${seconds}`;
}
```
调用函数示例:
```javascript
const date = new Date();
const formattedDate = formatDate(date);
console.log(formattedDate); // 输出格式为:2020-01-01 00:00:00
```
这样就可以通过该函数将 `new Date()` 类型的日期格式化为所需的格式。
### 回答3:
可以使用以下代码实现:
```javascript
function formatDate(date) {
const year = date.getFullYear();
const month = (date.getMonth() + 1).toString().padStart(2, '0');
const day = date.getDate().toString().padStart(2, '0');
const hours = date.getHours().toString().padStart(2, '0');
const minutes = date.getMinutes().toString().padStart(2, '0');
const seconds = date.getSeconds().toString().padStart(2, '0');
return `${year}-${month}-${day} ${hours}:${minutes}:${seconds}`;
}
const currentDate = new Date();
const formattedDate = formatDate(currentDate);
console.log(formattedDate); // 输出格式为:2020-01-01 00:00:00
```
在上述代码中,我们定义了一个名为`formatDate`的函数,接受一个`Date`类型的参数`date`。使用`date`对象的各个方法获取年、月、日、小时、分钟和秒的信息,并进行格式化。最后拼接这些信息,返回格式化后的日期字符串。
阅读全文
相关推荐
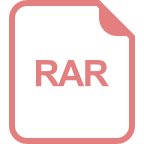















