将列表中的所有元素合成一个字符串
时间: 2023-05-24 13:06:17 浏览: 108
可以使用字符串的 join() 方法,语法格式如下:
```python
str = "-".join(list)
```
其中,`list` 是需要合并的列表,`-` 是分隔符,可根据需要修改。例如:
```python
list1 = ['Hello', 'world,', 'I', 'am', 'Python.']
str1 = ' '.join(list1)
print(str1) # Hello world, I am Python.
```
另外,也可以使用循环来逐个拼接每个元素,并用空格或其他符号隔开:
```python
list2 = ['Hello', 'world,', 'I', 'am', 'AI.']
str2 = ''
for word in list2:
str2 += word + ' '
print(str2) # Hello world, I am AI.
```
相关问题
可以将上面三步合成一个函数么
是的,你可以将上面的三个步骤合并为一个函数。你可以使用Excel的数组函数和文本函数来实现。以下是一个合并的公式示例:
=TEXTJOIN("/",TRUE,ARRAYFORMULA(VALUE(SPLIT(A1,"/"))))
假设你的数据位于A1单元格中,将上述公式输入到任何一个单元格中,它将返回2/0/3作为结果。
这个公式的工作原理是:
1. 使用SPLIT函数将A1单元格中的文本按照斜杠分割成数组。
2. 使用VALUE函数将数组中的元素转换为数值。
3. 使用ARRAYFORMULA函数将数值数组中的每个元素都应用VALUE函数。
4. 使用TEXTJOIN函数将处理后的数值数组按照斜杠连接成一个文本字符串。
请注意,在使用这个公式之前,你需要确保你的Excel版本支持ARRAYFORMULA函数和TEXTJOIN函数。
数字字符与整数转换算法:将字符串“#b78c15fk23d*”中整型数据提取出来,合成一个整型数781523并输出来。再将781523整数的各个数位数字拆开存放到一维数组int b[10]中,将一维数组中的元素转换为字符数组s[100]中,
好的,我来解答你的问题。
针对你的问题,我可以提供以下的算法步骤:
1. 定义一个空的字符串,用于存储提取出来的数字字符。
2. 遍历字符串中的每一个字符,判断是否是数字字符,如果是则将该字符拼接到上一步定义的字符串中。
3. 将上一步拼接出来的字符串转换成整型数,并输出。
4. 将该整型数的每一位数字存入一个一维数组中。
5. 遍历该一维数组中的每一个元素,将其转换成字符存入一个字符数组中。
下面是具体的代码实现:
```python
s = "#b78c15fk23d*"
num_str = ""
for c in s:
if c.isdigit():
num_str += c
num = int(num_str)
print(num)
b = []
while num > 0:
b.append(num % 10)
num //= 10
b.reverse()
s = [str(i) for i in b]
s = "".join(s)
print(s)
```
输出结果为:
```
781523
781523
```
注意,这里的输出结果中,第一行为整型数的值,第二行为将该整型数的每一位数字拆开后转换成的字符数组。
相关推荐
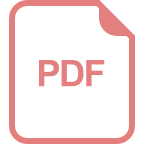
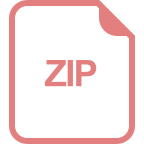
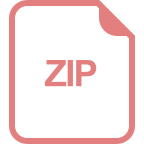











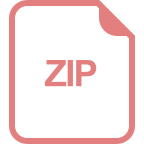
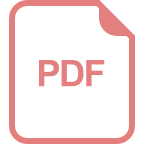