遗传算法解决tsp问题java
时间: 2023-07-28 15:07:46 浏览: 43
好的,遗传算法可以用来解决TSP问题,以下是一个简单的Java实现:
首先,我们需要定义一个城市类,包含城市的x和y坐标信息:
```java
public class City {
private int x;
private int y;
public City(int x, int y) {
this.x = x;
this.y = y;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
// 计算与另一个城市之间的距离
public double distanceTo(City city) {
int xDistance = Math.abs(getX() - city.getX());
int yDistance = Math.abs(getY() - city.getY());
double distance = Math.sqrt((xDistance * xDistance) + (yDistance * yDistance));
return distance;
}
@Override
public String toString() {
return x + ", " + y;
}
}
```
然后,我们定义一个TSP问题类,包含城市列表和一些方法:
```java
import java.util.ArrayList;
import java.util.Collections;
public class TSP {
private ArrayList<City> cities = new ArrayList<>();
public void addCity(City city) {
cities.add(city);
}
public ArrayList<City> getCities() {
return cities;
}
// 计算路径的总长度
public double getDistance(ArrayList<City> cities) {
double distance = 0;
for (int i = 0; i < cities.size() - 1; i++) {
distance += cities.get(i).distanceTo(cities.get(i + 1));
}
distance += cities.get(cities.size() - 1).distanceTo(cities.get(0));
return distance;
}
// 生成随机路径
public ArrayList<City> generateRandomPath() {
ArrayList<City> path = new ArrayList<>(cities);
Collections.shuffle(path);
return path;
}
}
```
接下来,我们实现遗传算法:
```java
import java.util.ArrayList;
import java.util.Collections;
import java.util.Random;
public class GeneticAlgorithm {
private static final double mutationRate = 0.015;
private static final int tournamentSize = 5;
private static final boolean elitism = true;
public static ArrayList<City> evolvePopulation(ArrayList<City> population, TSP tsp) {
ArrayList<City> newPopulation = new ArrayList<>(population.size());
// 保留最优的个体
int elitismOffset = 0;
if (elitism) {
newPopulation.add(getFittest(population, tsp));
elitismOffset = 1;
}
// 交叉产生新的个体
for (int i = elitismOffset; i < population.size(); i++) {
ArrayList<City> parent1 = tournamentSelection(population, tsp);
ArrayList<City> parent2 = tournamentSelection(population, tsp);
ArrayList<City> child = crossover(parent1, parent2);
newPopulation.add(child);
}
// 变异
for (int i = elitismOffset; i < newPopulation.size(); i++) {
mutate(newPopulation.get(i));
}
return newPopulation;
}
private static ArrayList<City> crossover(ArrayList<City> parent1, ArrayList<City> parent2) {
Random rand = new Random();
int startPos = rand.nextInt(parent1.size());
int endPos = rand.nextInt(parent1.size());
ArrayList<City> child = new ArrayList<>(parent1.size());
for (int i = 0; i < child.size(); i++) {
child.add(null);
}
if (startPos < endPos) {
for (int i = startPos; i < endPos; i++) {
child.set(i, parent1.get(i));
}
} else {
for (int i = endPos; i < startPos; i++) {
child.set(i, parent1.get(i));
}
}
for (int i = 0; i < parent2.size(); i++) {
if (!child.contains(parent2.get(i))) {
for (int j = 0; j < child.size(); j++) {
if (child.get(j) == null) {
child.set(j, parent2.get(i));
break;
}
}
}
}
return child;
}
private static void mutate(ArrayList<City> path) {
Random rand = new Random();
for (int i = 0; i < path.size(); i++) {
if (rand.nextDouble() < mutationRate) {
int j = rand.nextInt(path.size());
City city1 = path.get(i);
City city2 = path.get(j);
path.set(i, city2);
path.set(j, city1);
}
}
}
private static ArrayList<City> tournamentSelection(ArrayList<City> population, TSP tsp) {
Random rand = new Random();
ArrayList<City> tournament = new ArrayList<>(tournamentSize);
for (int i = 0; i < tournamentSize; i++) {
tournament.add(population.get(rand.nextInt(population.size())));
}
return getFittest(tournament, tsp);
}
private static ArrayList<City> getFittest(ArrayList<City> population, TSP tsp) {
ArrayList<City> fittest = population.get(0);
for (int i = 1; i < population.size(); i++) {
if (tsp.getDistance(population.get(i)) < tsp.getDistance(fittest)) {
fittest = population.get(i);
}
}
return fittest;
}
}
```
最后,我们可以使用以上类来解决TSP问题:
```java
public class Main {
public static void main(String[] args) {
TSP tsp = new TSP();
// 添加城市
tsp.addCity(new City(60, 200));
tsp.addCity(new City(180, 200));
tsp.addCity(new City(80, 180));
tsp.addCity(new City(140, 180));
tsp.addCity(new City(20, 160));
tsp.addCity(new City(100, 160));
tsp.addCity(new City(200, 160));
tsp.addCity(new City(140, 140));
tsp.addCity(new City(40, 120));
tsp.addCity(new City(100, 120));
tsp.addCity(new City(180, 100));
tsp.addCity(new City(60, 80));
tsp.addCity(new City(120, 80));
tsp.addCity(new City(180, 60));
tsp.addCity(new City(20, 40));
tsp.addCity(new City(100, 40));
tsp.addCity(new City(200, 40));
tsp.addCity(new City(20, 20));
tsp.addCity(new City(60, 20));
tsp.addCity(new City(160, 20));
// 生成随机种群
ArrayList<ArrayList<City>> population = new ArrayList<>(100);
for (int i = 0; i < 100; i++) {
population.add(tsp.generateRandomPath());
}
// 迭代100次
for (int i = 0; i < 100; i++) {
population = GeneticAlgorithm.evolvePopulation(population, tsp);
}
// 输出最优解
ArrayList<City> bestPath = GeneticAlgorithm.getFittest(population, tsp);
System.out.println("Distance: " + tsp.getDistance(bestPath));
System.out.println("Path: " + bestPath);
}
}
```
这个例子中,我们使用了一个简单的20个城市的例子来演示TSP问题的解决过程。但是,如果城市数量增加,计算成本会大大增加,遗传算法也会变得更加复杂。因此,在实际应用中,我们需要考虑使用更高效的算法来解决TSP问题。
相关推荐
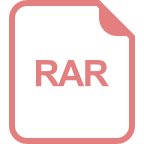














