python输出文件夹中png文件的路径
时间: 2024-03-23 09:43:30 浏览: 14
可以使用 Python 中的 os 模块和 glob 模块来实现这个功能。具体代码如下:
```python
import os
import glob
# 定义文件夹路径
folder_path = '/path/to/folder'
# 查找所有的 PNG 文件
png_files = glob.glob(os.path.join(folder_path, '*.png'))
# 输出每个 PNG 文件的路径
for png_file in png_files:
print(png_file)
```
在代码中,我们首先定义了一个文件夹路径 `folder_path`,然后使用 `glob.glob()` 函数查找该文件夹下所有的 PNG 文件。最后,使用循环输出每个 PNG 文件的路径。
相关问题
python将文件夹中png文件的绝对路径按cp936编码以文本形式输出到记事本中
可以使用Python的os和codecs库来实现。具体代码如下:
```python
import os
import codecs
# 文件夹路径,该路径下包含png文件
folder_path = 'your/folder/path'
# 获取文件夹中所有png文件的绝对路径
file_paths = [os.path.abspath(os.path.join(folder_path, f)) for f in os.listdir(folder_path) if f.endswith('.png')]
# 以cp936编码方式将文件路径写入文本文件
with codecs.open('file_paths.txt', 'w', 'cp936') as f:
f.write('\n'.join(file_paths))
```
将以上代码中的`your/folder/path`替换为你的文件夹路径,即可将该文件夹中所有png文件的绝对路径按cp936编码输出到名为`file_paths.txt`的文本文件中。
python删除文件夹中的png图像
你可以使用Python的os和glob模块来删除文件夹中的png图像。以下是一个示例代码:
```python
import os
import glob
# 设置文件夹路径
folder_path = "path/to/folder"
# 获取所有png文件的路径
png_files = glob.glob(os.path.join(folder_path, "*.png"))
# 遍历所有png文件的路径并删除它们
for file_path in png_files:
os.remove(file_path)
```
在这个示例代码中,我们首先使用`glob.glob()`函数获取指定文件夹中所有png格式的图像的路径。然后,我们使用`os.remove()`函数遍历每个路径并删除对应的文件。
相关推荐
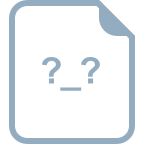
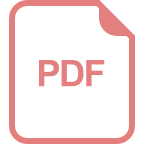
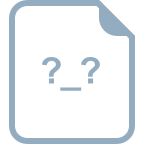












