在Linux编写C代码操作串口,网卡,音卡等设备,至少操作两种设备,给出代码
时间: 2024-01-17 11:03:33 浏览: 150
好的,下面我将分别给出Linux下C语言操作串口、网卡、音频设备的代码示例。
1. 操作串口
```c
#include <stdio.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
int main()
{
int fd;
struct termios opt;
// 打开串口设备
fd = open("/dev/ttyS0", O_RDWR);
if(fd == -1)
{
printf("Failed to open serial port!\n");
return -1;
}
// 设置串口属性
tcgetattr(fd, &opt);
opt.c_cflag = B115200 | CS8 | CLOCAL | CREAD;
opt.c_iflag = IGNPAR;
opt.c_oflag = 0;
opt.c_lflag = 0;
tcflush(fd, TCIFLUSH);
tcsetattr(fd, TCSANOW, &opt);
// 读写串口数据
char buf[256];
int len;
while(1)
{
len = read(fd, buf, 256);
if(len > 0)
{
write(fd, buf, len);
}
}
// 关闭串口设备
close(fd);
return 0;
}
```
2. 操作网卡
```c
#include <stdio.h>
#include <sys/socket.h>
#include <sys/ioctl.h>
#include <linux/if.h>
#include <netinet/in.h>
#include <arpa/inet.h>
int main()
{
int fd;
struct ifreq ifr;
struct sockaddr_in *addr;
// 创建socket
fd = socket(AF_INET, SOCK_DGRAM, 0);
if(fd == -1)
{
printf("Failed to create socket!\n");
return -1;
}
// 设置网卡名称和IP地址
strcpy(ifr.ifr_name, "eth0");
addr = (struct sockaddr_in *)&(ifr.ifr_addr);
addr->sin_family = AF_INET;
addr->sin_addr.s_addr = inet_addr("192.168.1.100");
ioctl(fd, SIOCSIFADDR, &ifr);
// 设置网卡子网掩码
addr->sin_addr.s_addr = inet_addr("255.255.255.0");
ioctl(fd, SIOCSIFNETMASK, &ifr);
// 设置网卡广播地址
addr->sin_addr.s_addr = inet_addr("192.168.1.255");
ioctl(fd, SIOCSIFBRDADDR, &ifr);
// 设置网卡MAC地址
unsigned char mac[] = {0x00, 0x11, 0x22, 0x33, 0x44, 0x55};
memcpy(ifr.ifr_hwaddr.sa_data, mac, 6);
ifr.ifr_hwaddr.sa_family = ARPHRD_ETHER;
ioctl(fd, SIOCSIFHWADDR, &ifr);
// 关闭socket
close(fd);
return 0;
}
```
3. 操作音频设备
```c
#include <stdio.h>
#include <stdlib.h>
#include <alsa/asoundlib.h>
int main()
{
int rc;
snd_pcm_t *handle;
snd_pcm_hw_params_t *params;
unsigned int val;
int dir;
snd_pcm_uframes_t frames;
char *buffer;
int size;
// 打开PCM设备
rc = snd_pcm_open(&handle, "default", SND_PCM_STREAM_PLAYBACK, 0);
if(rc < 0)
{
printf("Failed to open PCM device!\n");
return -1;
}
// 分配参数结构体
snd_pcm_hw_params_alloca(¶ms);
// 初始化参数结构体
snd_pcm_hw_params_any(handle, params);
// 设置参数
snd_pcm_hw_params_set_access(handle, params, SND_PCM_ACCESS_RW_INTERLEAVED);
snd_pcm_hw_params_set_format(handle, params, SND_PCM_FORMAT_S16_LE);
snd_pcm_hw_params_set_channels(handle, params, 2);
val = 44100;
snd_pcm_hw_params_set_rate_near(handle, params, &val, &dir);
frames = 32;
snd_pcm_hw_params_set_period_size_near(handle, params, &frames, &dir);
// 应用参数
rc = snd_pcm_hw_params(handle, params);
if(rc < 0)
{
printf("Failed to set PCM parameters!\n");
return -1;
}
// 计算缓冲区大小
snd_pcm_hw_params_get_period_size(params, &frames, &dir);
size = frames * 4;
buffer = (char *)malloc(size);
// 播放音频数据
while(1)
{
// 从音频文件读取数据
int len = fread(buffer, 1, size, stdin);
if(len <= 0)
{
break;
}
// 写入PCM设备
rc = snd_pcm_writei(handle, buffer, frames);
if(rc == -EPIPE)
{
snd_pcm_prepare(handle);
}
else if(rc < 0)
{
printf("Failed to write PCM data!\n");
}
}
// 关闭PCM设备
snd_pcm_close(handle);
free(buffer);
return 0;
}
```
以上三段代码分别展示了如何在Linux环境下使用C语言操作串口、网卡、音频设备。
阅读全文
相关推荐
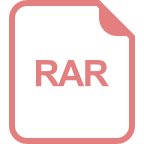

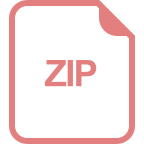
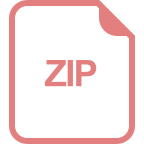
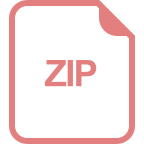
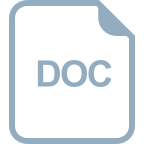
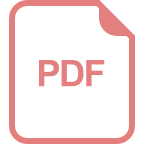
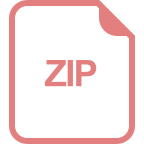
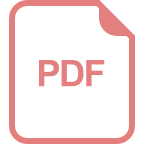
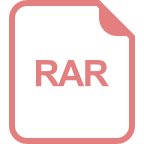
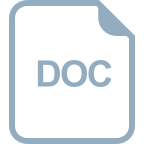
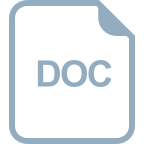
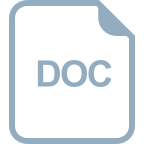
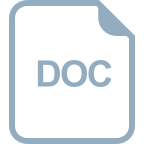
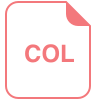