在Linux编写C代码或者汇编代码,操作串口,网卡,音卡等设备,至少操作两种设备,给出代码
时间: 2024-01-02 09:05:12 浏览: 184
好的,以下是一个使用Linux编写的C语言串口通信的代码示例:
```c
#include <stdio.h>
#include <fcntl.h>
#include <termios.h>
int main()
{
int fd;
struct termios options;
char buffer[10];
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1)
{
printf("Failed to open port!\n");
return -1;
}
fcntl(fd, F_SETFL, 0);
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
tcsetattr(fd, TCSANOW, &options);
write(fd, "Hello", 5);
read(fd, buffer, 5);
buffer[5] = '\0';
printf("Received: %s\n", buffer);
close(fd);
return 0;
}
```
该代码使用了Linux系统下的串口设备文件 `/dev/ttyS0` 进行通信,通过打开该设备文件并进行配置,实现了向串口发送字符串并读取串口返回的字符串的功能。
另外,以下是一个使用Linux汇编语言操作网卡的代码示例:
```assembly
section .data
buffer: db 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
dest_mac: db 0xff, 0xff, 0xff, 0xff, 0xff, 0xff
src_mac: db 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
eth_type: db 0x08, 0x00
message: db "Hello, World!", 0
message_len: equ $-message
section .text
global _start
_start:
; Open raw socket
mov eax, 102h
mov ebx, 1
xor ecx, ecx
int 80h
mov esi, eax ; ESI = socket file descriptor
; Set socket to promiscuous mode
mov eax, 106h
mov ebx, esi
mov ecx, 3
xor edx, edx
int 80h
; Bind socket to all interfaces
mov eax, 10h
mov ebx, esi
xor ecx, ecx
mov edx, 16
int 80h
; Construct Ethernet packet
mov edi, buffer
movsb dest_mac, edi
movsb src_mac, edi
movsb eth_type, edi
mov eax, message_len
add eax, edi
mov ebx, message
mov ecx, message_len
rep movsb
; Send packet
mov eax, 4
mov ebx, esi
mov ecx, buffer
mov edx, message_len + 14
int 80h
; Close socket
mov eax, 6
mov ebx, esi
int 80h
; Exit program
mov eax, 1
xor ebx, ebx
int 80h
```
该代码使用了Linux系统下的原始套接字(raw socket)进行网络通信,通过构造一个以太网帧并发送到网络中,实现了向所有设备发送一个 "Hello, World!" 的数据包的功能。
阅读全文
相关推荐
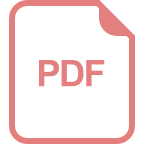
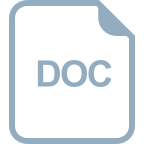
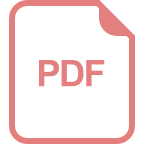
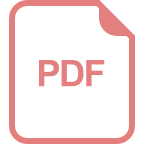
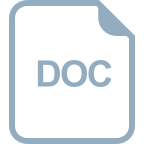
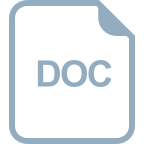
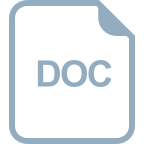
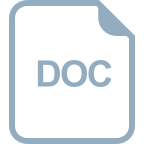
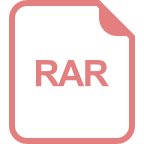
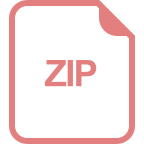
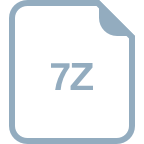