写一个java茶叶销售系统,链接数据库MYSQL,GUI程序
时间: 2023-08-16 08:08:03 浏览: 98
好的,以下是一个简单的Java茶叶销售系统的代码,使用了MySQL数据库和Swing GUI库。请注意,这只是一个示例,您可以根据自己的需求进行更改和调整。
首先,您需要设置MySQL数据库并创建一个名为“tea_sales”的数据库。在该数据库中,您需要创建一个名为“sales”的表格,具有以下字段:
- id (INT, PRIMARY KEY, AUTO_INCREMENT)
- name (VARCHAR(50))
- price (DOUBLE)
- quantity (INT)
- total (DOUBLE)
然后,您需要添加一些茶叶销售记录到该表中,以便程序可以显示和处理它们。
接下来,是Java代码:
```java
import java.sql.*;
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class TeaSalesSystem extends JFrame implements ActionListener {
JLabel nameLabel, priceLabel, quantityLabel, totalLabel;
JTextField nameField, priceField, quantityField, totalField;
JButton addButton, clearButton, refreshButton;
JTable salesTable;
JScrollPane scrollPane;
DefaultTableModel model;
public TeaSalesSystem() {
super("茶叶销售系统");
// 初始化GUI组件
nameLabel = new JLabel("茶叶名称:");
priceLabel = new JLabel("单价:");
quantityLabel = new JLabel("数量:");
totalLabel = new JLabel("总价:");
nameField = new JTextField(20);
priceField = new JTextField(10);
quantityField = new JTextField(10);
totalField = new JTextField(10);
totalField.setEditable(false);
addButton = new JButton("添加");
clearButton = new JButton("清空");
refreshButton = new JButton("刷新");
model = new DefaultTableModel();
salesTable = new JTable(model);
model.addColumn("ID");
model.addColumn("茶叶名称");
model.addColumn("单价");
model.addColumn("数量");
model.addColumn("总价");
scrollPane = new JScrollPane(salesTable);
// 设置GUI布局
JPanel inputPanel = new JPanel(new GridLayout(4, 2));
inputPanel.add(nameLabel);
inputPanel.add(nameField);
inputPanel.add(priceLabel);
inputPanel.add(priceField);
inputPanel.add(quantityLabel);
inputPanel.add(quantityField);
inputPanel.add(totalLabel);
inputPanel.add(totalField);
JPanel buttonPanel = new JPanel(new FlowLayout());
buttonPanel.add(addButton);
buttonPanel.add(clearButton);
buttonPanel.add(refreshButton);
setLayout(new BorderLayout());
add(inputPanel, BorderLayout.NORTH);
add(scrollPane, BorderLayout.CENTER);
add(buttonPanel, BorderLayout.SOUTH);
// 添加事件监听器
addButton.addActionListener(this);
clearButton.addActionListener(this);
refreshButton.addActionListener(this);
// 显示GUI界面
setSize(600, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == addButton) {
// 处理添加按钮事件
String name = nameField.getText();
String priceStr = priceField.getText();
String quantityStr = quantityField.getText();
if (name.isEmpty() || priceStr.isEmpty() || quantityStr.isEmpty()) {
JOptionPane.showMessageDialog(this, "请填写完整信息!", "错误", JOptionPane.ERROR_MESSAGE);
} else {
try {
double price = Double.parseDouble(priceStr);
int quantity = Integer.parseInt(quantityStr);
double total = price * quantity;
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost/tea_sales", "username", "password");
PreparedStatement stmt = conn.prepareStatement("INSERT INTO sales (name, price, quantity, total) VALUES (?, ?, ?, ?)");
stmt.setString(1, name);
stmt.setDouble(2, price);
stmt.setInt(3, quantity);
stmt.setDouble(4, total);
stmt.executeUpdate();
model.addRow(new Object[] { null, name, price, quantity, total });
salesTable.setModel(model);
nameField.setText("");
priceField.setText("");
quantityField.setText("");
totalField.setText("");
} catch (NumberFormatException ex) {
JOptionPane.showMessageDialog(this, "价格或数量格式不正确!", "错误", JOptionPane.ERROR_MESSAGE);
} catch (SQLException ex) {
JOptionPane.showMessageDialog(this, ex.getMessage(), "数据库错误", JOptionPane.ERROR_MESSAGE);
}
}
} else if (e.getSource() == clearButton) {
// 处理清空按钮事件
nameField.setText("");
priceField.setText("");
quantityField.setText("");
totalField.setText("");
} else if (e.getSource() == refreshButton) {
// 处理刷新按钮事件
try {
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost/tea_sales", "username", "password");
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM sales");
model.setRowCount(0);
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
double price = rs.getDouble("price");
int quantity = rs.getInt("quantity");
double total = rs.getDouble("total");
model.addRow(new Object[] { id, name, price, quantity, total });
}
salesTable.setModel(model);
nameField.setText("");
priceField.setText("");
quantityField.setText("");
totalField.setText("");
} catch (SQLException ex) {
JOptionPane.showMessageDialog(this, ex.getMessage(), "数据库错误", JOptionPane.ERROR_MESSAGE);
}
}
}
public static void main(String[] args) {
new TeaSalesSystem();
}
}
```
在上面的代码中,您需要将“username”和“password”替换为您的MySQL数据库的用户名和密码。
该程序中的GUI界面包括四个文本字段和三个按钮。每当用户单击“添加”按钮时,程序将从文本字段中读取茶叶的名称,单价和数量,并将它们插入到MySQL数据库的“sales”表中。程序还会计算总价并将其添加到表中。每当用户单击“清空”按钮时,程序将清除文本字段。每当用户单击“刷新”按钮时,程序将从MySQL数据库中检索所有现有的销售记录,并将它们添加到表中。
请注意,该程序仅是一个示例,您可以根据自己的需求进行更改和调整。
阅读全文
相关推荐
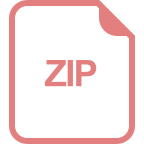
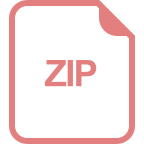
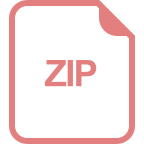
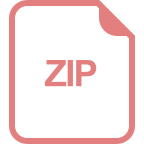
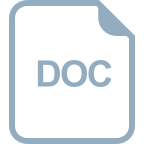
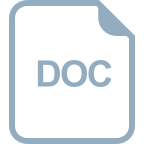
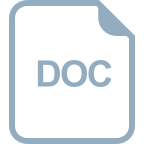
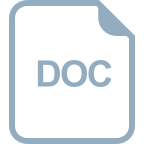
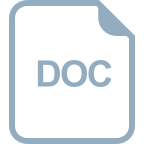
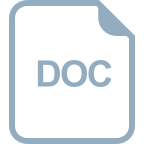
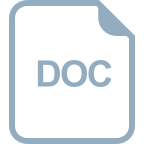
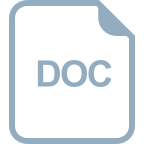
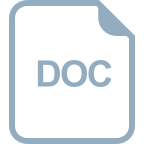
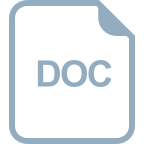
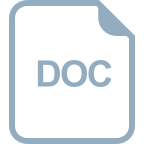
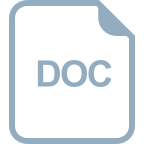
