php中使用接口的知识,定义一个形状,该形状包含面积计算公式和周长计算公式;分别定义圆形,矩形,三角形,都继承于该形状,都进行具体的面积和周长计算;
时间: 2024-03-23 10:41:53 浏览: 111
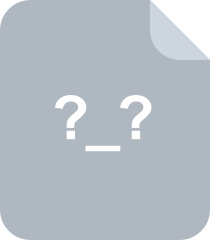
PHP计算矩形、三角形、圆形的面积及周长
好的,以下是实现代码:
```php
// 定义形状接口
interface Shape {
public function area();
public function perimeter();
}
// 定义圆形类
class Circle implements Shape {
private $radius;
public function __construct($radius) {
$this->radius = $radius;
}
public function area() {
return pi() * $this->radius * $this->radius;
}
public function perimeter() {
return 2 * pi() * $this->radius;
}
}
// 定义矩形类
class Rectangle implements Shape {
private $length;
private $width;
public function __construct($length, $width) {
$this->length = $length;
$this->width = $width;
}
public function area() {
return $this->length * $this->width;
}
public function perimeter() {
return 2 * ($this->length + $this->width);
}
}
// 定义三角形类
class Triangle implements Shape {
private $a;
private $b;
private $c;
public function __construct($a, $b, $c) {
$this->a = $a;
$this->b = $b;
$this->c = $c;
}
public function area() {
$s = ($this->a + $this->b + $this->c) / 2;
return sqrt($s * ($s - $this->a) * ($s - $this->b) * ($s - $this->c));
}
public function perimeter() {
return $this->a + $this->b + $this->c;
}
}
```
使用示例:
```php
// 创建圆形对象
$circle = new Circle(5);
echo "圆形的面积为:" . $circle->area() . "\n";
echo "圆形的周长为:" . $circle->perimeter() . "\n";
// 创建矩形对象
$rectangle = new Rectangle(4, 6);
echo "矩形的面积为:" . $rectangle->area() . "\n";
echo "矩形的周长为:" . $rectangle->perimeter() . "\n";
// 创建三角形对象
$triangle = new Triangle(3, 4, 5);
echo "三角形的面积为:" . $triangle->area() . "\n";
echo "三角形的周长为:" . $triangle->perimeter() . "\n";
```
输出结果:
```
圆形的面积为:78.539816339745
圆形的周长为:31.415926535898
矩形的面积为:24
矩形的周长为:20
三角形的面积为:6
三角形的周长为:12
```
阅读全文
相关推荐
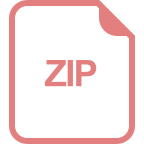
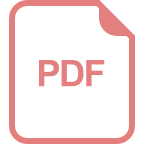



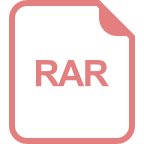
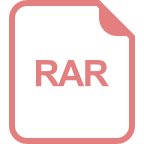
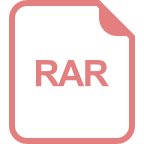
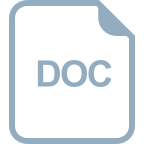

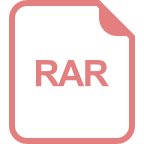
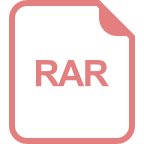
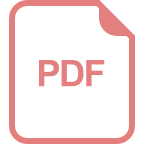
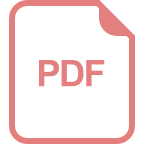
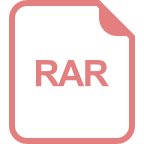
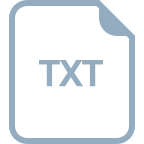
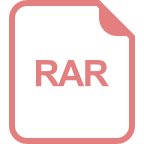