Qt5调用其他文件中的函数新建一个串口对象,怎么让我在这个文件中也能使用该串口对象
时间: 2023-12-10 21:38:44 浏览: 101
如果你在一个文件中创建了一个串口对象,并且想在另一个文件中使用该对象,可以将该对象作为参数传递给另一个文件中的函数。或者,你可以使用全局变量来存储该对象,并且在需要使用该对象的其他文件中将其声明为 extern。
以下是一个示例,假设你在 main.cpp 文件中创建了一个串口对象,你希望在另一个文件中使用该对象:
```c++
// main.cpp
#include "serial.h"
#include <QSerialPort>
int main(int argc, char *argv[])
{
QSerialPort serialPort;
// ...
Serial serial(serialPort); // 创建 Serial 类对象,并将串口对象作为参数传递
// ...
return 0;
}
```
```c++
// serial.h
#ifndef SERIAL_H
#define SERIAL_H
#include <QSerialPort>
class Serial
{
public:
Serial(QSerialPort &serialPort);
// ...
private:
QSerialPort &m_serialPort; // 成员变量保存串口对象的引用
};
#endif // SERIAL_H
```
```c++
// serial.cpp
#include "serial.h"
Serial::Serial(QSerialPort &serialPort)
: m_serialPort(serialPort)
{
// ...
}
```
现在,如果你需要在另一个文件中使用该串口对象,可以先在该文件中包含 serial.h 头文件,然后声明该对象为 extern:
```c++
// other_file.h
#ifndef OTHER_FILE_H
#define OTHER_FILE_H
#include "serial.h"
extern QSerialPort &serialPort; // 声明串口对象为 extern
void doSomethingWithSerialPort();
#endif // OTHER_FILE_H
```
在 other_file.cpp 文件中,你可以定义该对象,并使用它来调用 QSerialPort 类的函数:
```c++
// other_file.cpp
#include "other_file.h"
QSerialPort &serialPort = Serial::m_serialPort; // 定义串口对象并初始化为 Serial 类对象中的成员变量
void doSomethingWithSerialPort()
{
serialPort.write("Hello World"); // 使用串口对象调用函数
}
```
这样,在主文件中创建的串口对象就可以在其他文件中使用了。注意,你需要确保在使用该对象之前,主文件中的 Serial 类对象已经被实例化。
阅读全文
相关推荐
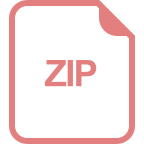
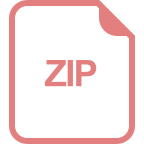





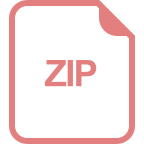
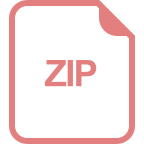





