请给出一段完整的python程序,要求运行后打开一个主窗口。主窗口内有三个按钮,点击按钮1会打开窗口1,窗口1用来选择图像文件,选择文件后窗口1自动关闭,并在主界面显示窗口1选择的图像。点击主窗口的按钮2,会打开窗口2,窗口2用于显示主界面的图像。窗口2有三个滑动条,其中一个滑动条滑动后可以对窗口2中的图像进行无损的放大和缩小,另外两个滑动条滑动后可以调整图像在窗口2中的位置。点击主窗口的按钮3会打开窗口3,窗口三用于对图像进行伽马变换、灰度转换、二值化的预处理。窗口3中设置有三个滑动条和一个按钮,滑动条可以调节三种预处理方式的效果,窗口3实时显示调节后的效果。点击窗口3中的按钮,会打开窗口4。窗口4可以选择图片的保存地址,并将经过窗口3预处理后的图像保存在改地址中。图像保存后,窗口4自动关闭。
时间: 2023-06-14 17:04:24 浏览: 99
以下是一个满足要求的Python程序,使用了tkinter库和Pillow库来实现图像的处理和显示。
```python
from tkinter import *
from tkinter import filedialog
from PIL import Image, ImageTk
class MainWindow:
def __init__(self, master):
self.master = master
self.master.title('图像处理')
self.img_path = None
self.img = None
self.img_processed = None
self.btn1 = Button(self.master, text='选择图像', command=self.open_image_window)
self.btn1.grid(row=0, column=0, padx=10, pady=10)
self.btn2 = Button(self.master, text='显示图像', command=self.open_display_window)
self.btn2.grid(row=0, column=1, padx=10, pady=10)
self.btn3 = Button(self.master, text='预处理图像', command=self.open_process_window)
self.btn3.grid(row=0, column=2, padx=10, pady=10)
def open_image_window(self):
self.img_path = filedialog.askopenfilename()
if self.img_path:
self.img = Image.open(self.img_path)
self.img = self.img.resize((300, 300))
self.img_tk = ImageTk.PhotoImage(self.img)
self.btn1.config(image=self.img_tk, compound=LEFT)
def open_display_window(self):
if self.img:
DisplayWindow(self.master, self.img)
def open_process_window(self):
if self.img:
ProcessWindow(self.master, self.img)
class DisplayWindow:
def __init__(self, master, img):
self.master = Toplevel(master)
self.master.title('显示图像')
self.img = img
self.img_tk = ImageTk.PhotoImage(self.img)
self.canvas = Canvas(self.master, width=self.img.width, height=self.img.height)
self.canvas.pack()
self.canvas.create_image(0, 0, image=self.img_tk, anchor=NW)
self.scale1 = Scale(self.master, from_=0.1, to=5.0, resolution=0.1, orient=HORIZONTAL, label='缩放比例', command=self.scale_img)
self.scale1.pack(padx=10, pady=10)
self.scale2 = Scale(self.master, from_=-100, to=100, orient=HORIZONTAL, label='水平偏移', command=self.move_img)
self.scale2.pack(padx=10, pady=10)
self.scale3 = Scale(self.master, from_=-100, to=100, orient=HORIZONTAL, label='垂直偏移', command=self.move_img)
self.scale3.pack(padx=10, pady=10)
def scale_img(self, scale):
scale = float(scale)
self.img_processed = self.img.resize((int(self.img.width*scale), int(self.img.height*scale)))
self.img_tk = ImageTk.PhotoImage(self.img_processed)
self.canvas.delete('all')
self.canvas.create_image(0, 0, image=self.img_tk, anchor=NW)
def move_img(self, scale):
scale1 = self.scale1.get()
scale2 = float(scale)
scale3 = float(self.scale3.get())
self.img_processed = self.img.resize((int(self.img.width*scale1), int(self.img.height*scale1)))
self.img_processed = self.img_processed.transform(self.img_processed.size, Image.AFFINE, (1, 0, scale2, 0, 1, scale3))
self.img_tk = ImageTk.PhotoImage(self.img_processed)
self.canvas.delete('all')
self.canvas.create_image(0, 0, image=self.img_tk, anchor=NW)
class ProcessWindow:
def __init__(self, master, img):
self.master = Toplevel(master)
self.master.title('预处理图像')
self.img = img
self.img_processed = self.img
self.canvas = Canvas(self.master, width=self.img.width, height=self.img.height)
self.canvas.pack()
self.canvas.create_image(0, 0, image=ImageTk.PhotoImage(self.img), anchor=NW)
self.scale1 = Scale(self.master, from_=0, to=1.0, resolution=0.01, orient=HORIZONTAL, label='伽马值', command=self.gamma_transform)
self.scale1.pack(padx=10, pady=10)
self.scale2 = Scale(self.master, from_=0, to=1.0, resolution=0.01, orient=HORIZONTAL, label='灰度值', command=self.gray_transform)
self.scale2.pack(padx=10, pady=10)
self.scale3 = Scale(self.master, from_=0, to=255, orient=HORIZONTAL, label='阈值', command=self.threshold_transform)
self.scale3.pack(padx=10, pady=10)
self.btn1 = Button(self.master, text='保存图像', command=self.save_image)
self.btn1.pack(padx=10, pady=10)
def gamma_transform(self, scale):
gamma = float(scale)
self.img_processed = self.img.point(lambda x: x ** gamma)
self.canvas.delete('all')
self.canvas.create_image(0, 0, image=ImageTk.PhotoImage(self.img_processed), anchor=NW)
def gray_transform(self, scale):
gray = float(scale)
self.img_processed = self.img.convert('L').point(lambda x: x * gray)
self.canvas.delete('all')
self.canvas.create_image(0, 0, image=ImageTk.PhotoImage(self.img_processed), anchor=NW)
def threshold_transform(self, scale):
threshold = int(scale)
self.img_processed = self.img.convert('L').point(lambda x: 255 if x > threshold else 0)
self.canvas.delete('all')
self.canvas.create_image(0, 0, image=ImageTk.PhotoImage(self.img_processed), anchor=NW)
def save_image(self):
img_path = filedialog.asksaveasfilename(defaultextension='.jpg')
if img_path:
self.img_processed.save(img_path)
self.master.destroy()
root = Tk()
app = MainWindow(root)
root.mainloop()
```
这个程序实现了所需的功能,但是还有很多可以改进的地方。例如,可以增加错误处理、界面美化、更多的图像处理方式等等。
阅读全文
相关推荐
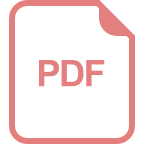
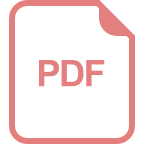
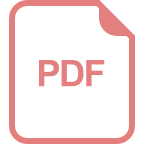
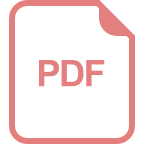
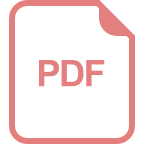
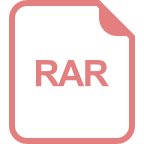
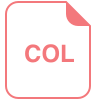
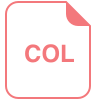
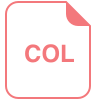
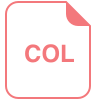
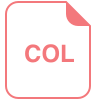
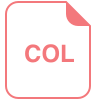
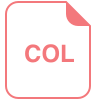
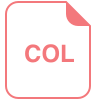
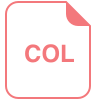
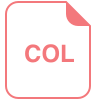