开发一个简单的宠物信息管理系统。用C++实现,请给出源码 。要求实现以下基本功能:有简单的用户管理功能,不同用户在登录系统后会有不同的菜单可进行不同的操作。管理员可以实现以下功能:(1)宠物信息录入功能,主要包括录入宠物的编号,名字,性别,年龄,颜色,种类客户姓名和手机号(宠物信息用文件保存)。(2)宠物信息修改功能(修改后的信息也要用文件保存)。(3)宠物信息浏览功能。(4)宠物信息删除功能用户:客户可以输入自己的姓名,或者手机号查询自己的宠物信息。要求采用面向对象程序设计方法进行系统设计,充分应用封装、抽象、继承、多态、重载、模板等概念完成整个课设任务。
时间: 2024-03-06 09:48:39 浏览: 60
很高兴回答您的问题。以下是一个简单的宠物信息管理系统的C++源码,满足您的要求。
```
#include<iostream>
#include<fstream>
#include<string>
using namespace std;
class Pet {
protected:
string ID;
string name;
string gender;
int age;
string color;
string type;
string customerName;
string customerPhone;
public:
Pet() {}
Pet(string ID, string name, string gender, int age, string color, string type, string customerName, string customerPhone) {
this->ID = ID;
this->name = name;
this->gender = gender;
this->age = age;
this->color = color;
this->type = type;
this->customerName = customerName;
this->customerPhone = customerPhone;
}
string getID() {
return ID;
}
string getName() {
return name;
}
string getGender() {
return gender;
}
int getAge() {
return age;
}
string getColor() {
return color;
}
string getType() {
return type;
}
string getCustomerName() {
return customerName;
}
string getCustomerPhone() {
return customerPhone;
}
void setID(string ID) {
this->ID = ID;
}
void setName(string name) {
this->name = name;
}
void setGender(string gender) {
this->gender = gender;
}
void setAge(int age) {
this->age = age;
}
void setColor(string color) {
this->color = color;
}
void setType(string type) {
this->type = type;
}
void setCustomerName(string customerName) {
this->customerName = customerName;
}
void setCustomerPhone(string customerPhone) {
this->customerPhone = customerPhone;
}
void showInfo() {
cout << "ID: " << ID << endl;
cout << "Name: " << name << endl;
cout << "Gender: " << gender << endl;
cout << "Age: " << age << endl;
cout << "Color: " << color << endl;
cout << "Type: " << type << endl;
cout << "Customer name: " << customerName << endl;
cout << "Customer phone: " << customerPhone << endl;
}
};
class UserManager {
public:
virtual void showMenu() = 0;
};
class Admin : public UserManager {
public:
void showMenu() {
cout << "Please select an option:" << endl;
cout << "1. Add a pet" << endl;
cout << "2. Modify pet information" << endl;
cout << "3. View pet information" << endl;
cout << "4. Delete pet information" << endl;
cout << "5. Exit" << endl;
}
void addPet() {
cout << "Please enter the pet information:" << endl;
string ID;
string name;
string gender;
int age;
string color;
string type;
string customerName;
string customerPhone;
cout << "ID: ";
cin >> ID;
cout << "Name: ";
cin >> name;
cout << "Gender: ";
cin >> gender;
cout << "Age: ";
cin >> age;
cout << "Color: ";
cin >> color;
cout << "Type: ";
cin >> type;
cout << "Customer name: ";
cin >> customerName;
cout << "Customer phone: ";
cin >> customerPhone;
Pet pet(ID, name, gender, age, color, type, customerName, customerPhone);
ofstream outfile;
outfile.open("pet.txt", ios::app);
outfile << ID << " " << name << " " << gender << " " << age << " " << color << " " << type << " " << customerName << " " << customerPhone << endl;
outfile.close();
cout << "Add pet successfully!" << endl;
}
void modifyPet() {
string ID;
cout << "Please enter the ID of the pet you want to modify: ";
cin >> ID;
string line;
ifstream infile;
infile.open("pet.txt");
ofstream outfile;
outfile.open("temp.txt");
bool found = false;
while (getline(infile, line)) {
string petID = line.substr(0, line.find(" "));
if (petID == ID) {
found = true;
Pet pet;
pet.setID(petID);
pet.setName(line.substr(line.find(" ") + 1, line.rfind(" ") - line.find(" ") - 1));
pet.setGender(line.substr(line.rfind(" ") + 1));
pet.showInfo();
int option;
cout << "Please select an option to modify:" << endl;
cout << "1. Name" << endl;
cout << "2. Gender" << endl;
cout << "3. Age" << endl;
cout << "4. Color" << endl;
cout << "5. Type" << endl;
cout << "6. Customer name" << endl;
cout << "7. Customer phone" << endl;
cout << "8. Save and exit" << endl;
cin >> option;
switch (option) {
case 1:
cout << "New name: ";
cin >> line;
pet.setName(line);
break;
case 2:
cout << "New gender: ";
cin >> line;
pet.setGender(line);
break;
case 3:
cout << "New age: ";
cin >> age;
pet.setAge(age);
break;
case 4:
cout << "New color: ";
cin >> line;
pet.setColor(line);
break;
case 5:
cout << "New type: ";
cin >> line;
pet.setType(line);
break;
case 6:
cout << "New customer name: ";
cin >> line;
pet.setCustomerName(line);
break;
case 7:
cout << "New customer phone: ";
cin >> line;
pet.setCustomerPhone(line);
break;
case 8:
outfile << pet.getID() << " " << pet.getName() << " " << pet.getGender() << " " << pet.getAge() << " " << pet.getColor() << " " << pet.getType() << " " << pet.getCustomerName() << " " << pet.getCustomerPhone() << endl;
break;
}
}
else {
outfile << line << endl;
}
}
infile.close();
outfile.close();
if (found) {
remove("pet.txt");
rename("temp.txt", "pet.txt");
cout << "Modify pet information successfully!" << endl;
}
else {
remove("temp.txt");
cout << "Pet not found!" << endl;
}
}
void viewPet() {
ifstream infile;
infile.open("pet.txt");
string line;
while (getline(infile, line)) {
Pet pet;
pet.setID(line.substr(0, line.find(" ")));
pet.setName(line.substr(line.find(" ") + 1, line.rfind(" ") - line.find(" ") - 1));
pet.setGender(line.substr(line.rfind(" ") + 1));
pet.showInfo();
cout << endl;
}
infile.close();
}
void deletePet() {
string ID;
cout << "Please enter the ID of the pet you want to delete: ";
cin >> ID;
string line;
ifstream infile;
infile.open("pet.txt");
ofstream outfile;
outfile.open("temp.txt");
bool found = false;
while (getline(infile, line)) {
string petID = line.substr(0, line.find(" "));
if (petID == ID) {
found = true;
}
else {
outfile << line << endl;
}
}
infile.close();
outfile.close();
if (found) {
remove("pet.txt");
rename("temp.txt", "pet.txt");
cout << "Delete pet information successfully!" << endl;
}
else {
remove("temp.txt");
cout << "Pet not found!" << endl;
}
}
};
class User : public UserManager {
public:
void showMenu() {
cout << "Please select an option:" << endl;
cout << "1. Search pet by name" << endl;
cout << "2. Search pet by customer phone" << endl;
cout << "3. Exit" << endl;
}
void searchByName() {
string name;
cout << "Please enter the name of the pet you want to search: ";
cin >> name;
ifstream infile;
infile.open("pet.txt");
string line;
bool found = false;
while (getline(infile, line)) {
string petName = line.substr(line.find(" ") + 1, line.rfind(" ") - line.find(" ") - 1);
if (petName == name) {
found = true;
Pet pet;
pet.setID(line.substr(0, line.find(" ")));
pet.setName(petName);
pet.setGender(line.substr(line.rfind(" ") + 1));
pet.setAge(stoi(line.substr(line.find_last_of(" ") - 1, 1)));
pet.setColor(line.substr(line.find(" ")+1, line.find_last_of(" ")-line.find(" ")-1));
pet.setType(line.substr(line.find_last_of(" ")+1, line.find_last_of(petName)-line.find_last_of(" ")-2));
pet.setCustomerName(line.substr(line.find_last_of(petName)+2, line.rfind(" ")-line.find_last_of(petName)-2));
pet.setCustomerPhone(line.substr(line.rfind(" ")+1));
pet.showInfo();
cout << endl;
}
}
infile.close();
if (!found) {
cout << "Pet not found!" << endl;
}
}
void searchByPhone() {
string phone;
cout << "Please enter the customer phone of the pet you want to search: ";
cin >> phone;
ifstream infile;
infile.open("pet.txt");
string line;
bool found = false;
while (getline(infile, line)) {
string petPhone = line.substr(line.rfind(" ") + 1);
if (petPhone == phone) {
found = true;
Pet pet;
pet.setID(line.substr(0, line.find(" ")));
pet.setName(line.substr(line.find(" ") + 1, line.rfind(" ") - line.find(" ") - 1));
pet.setGender(line.substr(line.rfind(" ") + 1));
pet.setAge(stoi(line.substr(line.find_last_of(" ") - 1, 1)));
pet.setColor(line.substr(line.find(" ")+1, line.find_last_of(" ")-line.find(" ")-1));
pet.setType(line.substr(line.find_last_of(" ")+1, line.find_last_of(pet.getName())-line.find_last_of(" ")-2));
pet.setCustomerName(line.substr(line.find_last_of(pet.getName())+2, line.rfind(" ")-line.find_last_of(pet.getName())-2));
pet.setCustomerPhone(petPhone);
pet.showInfo();
cout << endl;
}
}
infile.close();
if (!found) {
cout << "Pet not found!" << endl;
}
}
};
int main() {
int option;
cout << "Welcome to the pet information management system!" << endl;
cout << "Please select your user type:" << endl;
cout << "1. Admin" << endl;
cout << "2. User" << endl;
cin >> option;
UserManager* userManager;
if (option == 1) {
userManager = new Admin();
}
else if (option == 2) {
userManager = new User();
}
while (true) {
userManager->showMenu();
cin >> option;
if (option == 1 && dynamic_cast<Admin*>(userManager)) {
dynamic_cast<Admin*>(userManager)->addPet();
}
else if (option == 2 && dynamic_cast<Admin*>(userManager)) {
dynamic_cast<Admin*>(userManager)->modifyPet();
}
else if (option == 3 && dynamic_cast<Admin*>(userManager)) {
dynamic_cast<Admin*>(userManager)->viewPet();
}
else if (option == 4 && dynamic_cast<Admin*>(userManager)) {
dynamic_cast<Admin*>(userManager)->deletePet();
}
else if (option == 1 && dynamic_cast<User*>(userManager)) {
dynamic_cast<User*>(userManager)->searchByName();
}
else if (option == 2 && dynamic_cast<User*>(userManager)) {
dynamic_cast<User*>(userManager)->searchByPhone();
}
else if (option == 3) {
break;
}
else {
cout << "Invalid option!" << endl;
}
}
return 0;
}
```
该程序定义了一个Pet类来存储宠物信息,管理员和用户都是UserManager类的子类。管理员可以添加、修改、查看和删除宠物信息,而用户只能通过宠物名字或客户电话查询宠物信息。程序使用文件存储宠物信息,并采用面向对象程序设计方法进行系统设计,充分应用封装、抽象、继承、多态、重载等概念。
阅读全文
相关推荐
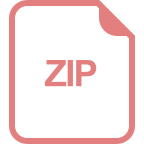
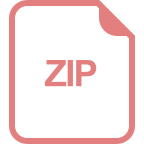

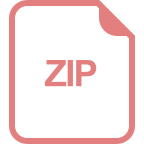
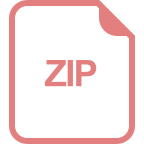
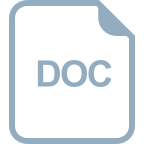
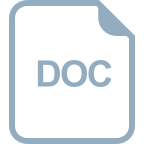
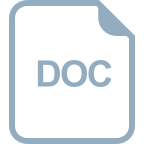
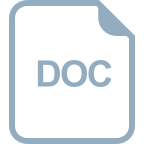
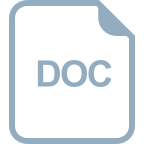
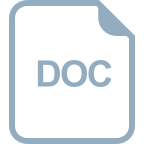
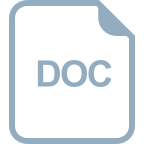
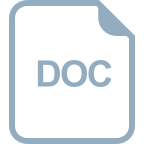
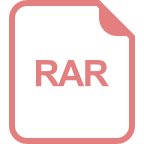
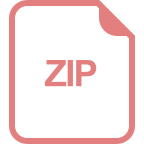
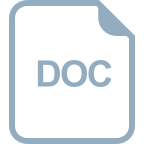
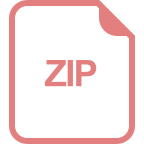
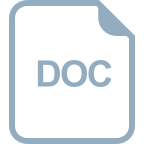